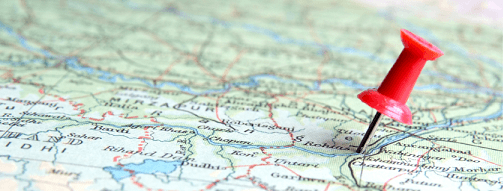
HTML5 has finally pushed the web ahead with many new APIs that were lacking since the inception of HTML. New APIs like DOM Storage API helps in making better web applications. As part of the specification, HTML5 added set of new APIs under title Geolocation that helps the applications to identify location related information from visiting users. Here is the official definition from HTML5 specification:
The Geolocation API defines a high-level interface to location information associated only with the device hosting the implementation, such as latitude and longitude. The API itself is agnostic of the underlying location information sources. Common sources of location information include Global Positioning System (GPS) and location inferred from network signals such as IP address, RFID, WiFi and Bluetooth MAC addresses, and GSM/CDMA cell IDs, as well as user input. No guarantee is given that the API returns the device’s actual location.
The API can be used to get current location (latitude, longitude) of user.
Understanding Geolocation API
In order to utilize Geolocation API in a web application, we must understand the API structures. The browser that supports Geolocation API provides navigator object with geolocation
object.This object can be used to retrieve location info. Following are the methods:
void getCurrentPosition(PositionCallback successCallback,
optional PositionErrorCallback errorCallback,
optional PositionOptions options);
Code language: JavaScript (javascript)
This method takes a callback method successCallback which gets calledonce the location of user (browser) is determined. The geolocation object provides following useful methods to retrieve location related information.
interface Geolocation {
void getCurrentPosition(PositionCallback successCallback,
optional PositionErrorCallback errorCallback,
optional PositionOptions options);
long watchPosition(PositionCallback successCallback,
optional PositionErrorCallback errorCallback,
optional PositionOptions options);
void clearWatch(long watchId);
};
//watchPosition() method will register a handler which repeteadly
// gets called with current location details.
// callback PositionCallback = void (Position position);
// callback PositionErrorCallback = void (PositionError positionError);
Code language: JavaScript (javascript)
Getting Current Location
Following is the simple example to retrieve current location of user’s browser. Note that your browser must support geolocation in order to run this example.
HTML Code
First we define a plain HTML, a button called “Show Location” and two spans to display latitude and longitude.
<html>
...
<input type="button" value="Show Location"
onclick="javascript:showlocation()" /> <br/>
Latitude: <span id="latitude"></span> <br/>
Longitude: <span id="longitude"></span>
...
</html>
Code language: HTML, XML (xml)
JavaScript Code
In below javascript we defined a function showlocation() that gets called on click of “Show Location” button. This method registers a callback function called “callback” which does the work of displaying the latitude and longitude.
function showlocation() {
// One-shot position request.
navigator.geolocation.getCurrentPosition(callback);
}
function callback(position) {
document.getElementById('latitude').innerHTML = position.coords.latitude;
document.getElementById('longitude').innerHTML = position.coords.longitude;
}
Code language: JavaScript (javascript)
Demo
Geolocation API and Google Maps integration
Let us quickly check a demo where we integrate HTML5 Geolocation API and Google Maps API. The idea is to fetch current location of user using Geolocation API and then showing it on map. Check out the demo first before we dig into the code.
Demo
Following is the complete code snippet. See how we adapted above code. The showlocation()
method is called when the button is clicked. It initialize the Geolocation API using navigator.geolocation.getCurrentPosition()
method. A callback method callback() is registered which gets called once the location is retrieved. In callback() method we fetch the latitude and longitude and set the Google map its center.
<!DOCTYPE html>
<html>
<head>
<script type="text/javascript"
src="https://maps.googleapis.com/maps/api/js?sensor=false">
</script>
<script type="text/javascript">
var map = null;
function showlocation() {
// One-shot position request.
navigator.geolocation.getCurrentPosition(callback);
}
function callback(position) {
var lat = position.coords.latitude;
var lon = position.coords.longitude;
document.getElementById('latitude').innerHTML = lat;
document.getElementById('longitude').innerHTML = lon;
var latLong = new google.maps.LatLng(lat, lon);
var marker = new google.maps.Marker({
position: latLong
});
marker.setMap(map);
map.setZoom(8);
map.setCenter(marker.getPosition());
}
google.maps.event.addDomListener(window, 'load', initMap);
function initMap() {
var mapOptions = {
center: new google.maps.LatLng(0, 0),
zoom: 1,
mapTypeId: google.maps.MapTypeId.ROADMAP
};
map = new google.maps.Map(document.getElementById("map-canvas"),
mapOptions);
}
</script>
</head>
<body>
<center>
<input type="button" value="Show my location on Map"
onclick="javascript:showlocation()" /> <br/>
</center>
Latitude: <span id="latitude"></span> <br/>
Longitude: <span id="longitude"></span>
<br/><br/>
<div id="map-canvas"/>
</body>
</html>
Code language: HTML, XML (xml)
Error Handling
There are different error conditions that one should take care while dealing with Geolocation API. The second parameter of the getCurrentPosition()
method is used to handle errors. It specifies a function to run if it fails to get the user’s location:
Code language: JavaScript (javascript)navigator.geolocation.getCurrentPosition(callback, errorHandler);
The errorHandler() method is called if there is any error while retrieving user location. This method will be called with one argument: error code. The error code should be checked for following values to know what type of error occurred.
Error code | Meaning |
---|---|
PERMISSION_DENIED | User denied the request for Geolocation. |
POSITION_UNAVAILABLE | Location information is unavailable. |
TIMEOUT | The request to get user location timed out. |
UNKNOWN_ERROR | An unknown error occurred. |
Source code
//..
navigator.geolocation.getCurrentPosition(callback, errorHandler);
//..
function errorHandler(error) {
switch (error.code) {
case error.PERMISSION_DENIED:
alert("User denied the request for Geolocation.");
break;
case error.POSITION_UNAVAILABLE:
alert("Location information is unavailable.");
break;
case error.TIMEOUT:
alert("The request to get user location timed out.");
break;
case error.UNKNOWN_ERROR:
alert("An unknown error occurred.");
break;
}
}
Code language: JavaScript (javascript)
Get Current Location Repeatedly (Watching the location)
We saw how to get current user location using getCurrentPosition() method. However this method only gives location only once, when it is called. Sometimes it is desirable to get users location continuously if it changes. This will never happen if user is accessing webpage from a desktop machine. But smartphones/tablets are common contenders to use Geolocation API and get location continuously if user is on move. The method watchPosition() is used to watch users location. Below is the syntax for this method:
Code language: JavaScript (javascript)long navigator.geolocation.watchPosition(callback);
The method takes one argument, the callback function which gets called when location is obtained. Note that unlike getCurrentPosition()
method, the watchPosition()
method returns a long value watchID. This id can be used to uniquely identify the requested position watcher; and this value can be used in tandem with the clearPosition()
method to stop watching the user’s location.
Code language: JavaScript (javascript)navigator.geolocation.clearWatch(watchID);
Check out the demo below. You need to open this demo in device that has GPS built-in. Otherwise this won’t work. Try opening this page in phone/tablet with GPS and move around. The latitude / longitude should change while you on move :)
Demo
Source code
<!DOCTYPE html>
<html>
<body>
Click Show location button and run :)
<input type="button" value="Show Location" onclick="showlocation()" />
<br />
Latitude: <span id="latitude">..</span> <br />
Longitude: <span id="longitude">..</span>
<script>
function showlocation() {
navigator.geolocation.watchPosition(callback);
}
function callback(position) {
document.getElementById("latitude").innerHTML = position.coords.latitude;
document.getElementById("longitude").innerHTML = position.coords.longitude;
}
</script>
</body>
</html>
Code language: HTML, XML (xml)
Browser compatibility
Like all other HTML5 APIs, the Geolocation API is also new in the town and not all browsers supports it. Following are the list of browsers with minimum version that have support for this API.
Chrome | Firefox (Gecko) | Internet Explorer | Opera | Safari |
---|---|---|---|---|
5 | 3.5 (1.9.1) | 9 | 10.60 Removed in 15.0 Reintroduced in 16.0 | 5 |
I hope this is useful. Let me know if you using HTML5 Geolocation API in some creative way. Would be interesting to know different use cases for this API :)
I really like this on a blog I’m tweeting. U Rock!
I am using above code to show current location geo coordinates in dream weaver.. Its not working for me…Could you please help me to solve this problem
Click the “Allow” button of the pop-up , otherwise it will not work.
Very Nice Article..
This post is helpful, thanks !
-www.77-thoughts.com
Very nice article, thanks a lot…
Is there any solution for Safari Browser. In my case working for all browser left Safari. Please Help me out ……….
if you have problem view the map..please insert this CSS code
html, body, #map-canvas {
height: 200px;
margin: 15px;
padding: 0px
}
Hi Viral,
how to capture user destination location using map?
sai
Hice article thanks, but why <input type="text" or <input id="blabla" or even input class="field" way doesn't work? Do you have any idea for fix tis?
try the Code Below for current location!
Hi ,
if i run this HTML code my Map is not loaded ?
can u please send me the source code showing the current locatino in map please !! this is my email id:[email protected]
Hello, using the code i am able to get the longitude, and latitude, which i am storing into a database. But at the same time i want to save City, District, State into respective fields. Can it possible using java script. Thanks in advance.
I have implemented Html5 geo-location.But its not working in mobile Devices if the location services is tuned off.
However , I am connected with WIFI .its not pin pointing my location
sir only show the lat and lon but did’t shown the map plzz help me…the map is not visible but lan,log is visible
Amazing, I googled info about that and I found that you have a website! Keep it Plastic!
Hi guys
This geolocation api returns lon and lat of center of city, not exactly my current location because there is big gap between me and center of my city(almost 2 – 3 km). So is there any means or how can i get lat and lon of my current location / street.
Best
Could you please let me know how we can show more than one device location in the map?
I realize I’m asking a very, very basic question, but I’m having trouble getting past step one in learning Google Script. I open Google Drive, I create a new Google Apps Script. I use this code:
And I get an error stating: ReferenceError: “navigator” is not defined. (line 3, file “Code”)
How do I use these code examples? The web is full of great examples, but I have gotten a grand total of zero to work. What am I missing?
Hi,
I have a code with a check box to enable the user to show/hide his current location
the code works fine showing the blue circle at current location on the map
however I cant seem to be able to hide it or disable shwoing of the blue circle
In the code GeoMarker is define golobally, all attempts to set map to null is not working, need any help with that. Thanks
Hi
Nice tutorial. Can you please post a tutorial where one imports a kml file from a third party server say a bicycle trail and one can also see his location at that moment when he is following the trail.Can we use the google map api v3 ? can this be done ?
I would like to access only satelite view is there any source code API to acess it.
Amazing, I googled info about that and I found that you have a website! Keep it Plastic!
Reply
guru 25 July, 2014, 19:11
Hi guys
This geolocation api returns lon and lat of center of city, not exactly my current location because there is big gap between me and center of my city(almost 2 – 3 km). So is there any means or how can i get lat and lon of my current location / street.
Best
Reply
Mahsa 15 August, 2014, 5:28
Could you please let me know how we can show more than one device location in the map?
Reply
John Mayson 22 September, 2014, 9:26
I realize I’m asking a very, very basic question, but I’m having trouble getting past step one in learning Google Script. I open Google Drive, I create a new Google Apps Script. I use this code:
function showlocation() {
// One-shot position request.
navigator.geolocation.getCurrentPosition(callback);
}
function callback(position) {
document.getElementById(‘latitude’).innerHTML = position.coords.latitude;
document.getElementById(‘longitude’).innerHTML = position.coords.longitude;
}
And I get an error stating: ReferenceError: “navigator” is not defined. (line 3, file “Code”)
How do I use these code examples? The web is full of great examples, but I have gotten a grand total of zero to work. What am I missing?
Reply
Ahmed 14 October, 2014, 6:14
Hi,
I have lat and lag of current locatio but how can i convert it in readable address , country name or city nae…
I am running the source code using google chrome Version 56.0.2924.87. Only latitude and longitude coordinates are displayed but map is not displayed.What I have to do? Can anyone resolve this issue ?
Its not working with websites who doesn’t have HTTPS.