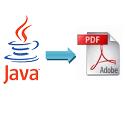
iText is very powerful library. It is used extensively to create PDF files in Java applications. Although not free, iText is the de-fecto Java library for working with PDF files. It has been extensively used in different Java related products for generating and manipulating PDF files. Let us see how iText can be used to set password protection (encryption) to a PDF file. Also how to read an encrypted PDF file in Java. For this we are using our Generate PDF in Java using iText tutorial. You might want to have a look into that tutorial to have an overview of iText and its different APIs that can be used for PDF generation. To set encryption in iText following API can be used.
String USER_PASS = "Hello123";
String OWNER_PASS = "Owner123";
PdfWriter writer = PdfWriter.getInstance(document, file);
writer.setEncryption(USER_PASS.getBytes(), OWNER_PASS.getBytes(),
PdfWriter.ALLOW_PRINTING, PdfWriter.ENCRYPTION_AES_128);
Code language: Java (java)
Thus .setEncryption()
method sets password protection to any PDF file. Before we get started with code, we need few JAR files. iText internally uses bouncycastle.org library to encrypt the PDF files. We will need following JAR files in addition to iText JAR.
- itextpdf-5.2.1.jar
- bcmail-jdk16-1.46.jar
- bcprov-jdk16-1.46.jar
- bctsp-jdk16-1.46.jar
You can download all these JARs along with the demo source code at the end of this tutorial. Now following is the Java code to Generate a PDF file and set Password protection to it.
package net.viralpatel.pdf;
import java.io.File;
import java.io.FileOutputStream;
import java.io.OutputStream;
import java.util.Date;
import com.itextpdf.text.Document;
import com.itextpdf.text.Paragraph;
import com.itextpdf.text.pdf.PdfWriter;
public class GeneratePDF {
private static String USER_PASS = "Hello123";
private static String OWNER_PASS = "Owner123";
public static void main(String[] args) {
try {
OutputStream file = new FileOutputStream(new File("D:\\Test.pdf"));
Document document = new Document();
PdfWriter writer = PdfWriter.getInstance(document, file);
writer.setEncryption(USER_PASS.getBytes(), OWNER_PASS.getBytes(),
PdfWriter.ALLOW_PRINTING, PdfWriter.ENCRYPTION_AES_128);
document.open();
document.add(new Paragraph("Hello World, iText"));
document.add(new Paragraph(new Date().toString()));
document.close();
file.close();
} catch (Exception e) {
e.printStackTrace();
}
}
}
Code language: Java (java)
Thus we used the same steps to generate PDF file that we used in previous tutorial. Just added one line writer.setEncryption()
to set password to generated PDF. Note that in setEncryption() method, we passes several arguments. First two parameters are the password values in bytes. We passed Hello123 and Owner123 as password values. Also the next argument is permission you want to give to user. Following are several permission values.
Code language: Java (java)PdfWriter.ALLOW_PRINTING PdfWriter.ALLOW_ASSEMBLY PdfWriter.ALLOW_COPY PdfWriter.ALLOW_DEGRADED_PRINTING PdfWriter.ALLOW_FILL_IN PdfWriter.ALLOW_MODIFY_ANNOTATIONS PdfWriter.ALLOW_MODIFY_CONTENTS PdfWriter.ALLOW_SCREENREADERS
You can provide multiple permissions by ORing different values. For example PdfWriter.ALLOW_PRINTING | PdfWriter.ALLOW_COPY
. The last parameter is the type of encryption you want to use to encrypt PDF. Following are several allowed values.
Code language: Java (java)PdfWriter.ENCRYPTION_AES_128 PdfWriter.ENCRYPTION_AES_256
Download Source Code
Java_iText_PDF_Password.zip (8.9 MB)
Hi viralpatel,i tried the example but i got the Following Error at RunTime
java.lang.SecurityException: class “org.bouncycastle.asn1.ASN1ObjectIdentifier”‘s signer information does not match signer information of other classes in the same package
at java.lang.ClassLoader.checkCerts(ClassLoader.java:776)
at java.lang.ClassLoader.preDefineClass(ClassLoader.java:488)
at java.lang.ClassLoader.defineClass(ClassLoader.java:615)
at java.security.SecureClassLoader.defineClass(SecureClassLoader.java:124)
at java.net.URLClassLoader.defineClass(URLClassLoader.java:260)
at java.net.URLClassLoader.access$100(URLClassLoader.java:56)
at java.net.URLClassLoader$1.run(URLClassLoader.java:195)
at java.security.AccessController.doPrivileged(Native Method)
at java.net.URLClassLoader.findClass(URLClassLoader.java:188)
at java.lang.ClassLoader.loadClass(ClassLoader.java:307)
at sun.misc.Launcher$AppClassLoader.loadClass(Launcher.java:268)
at java.lang.ClassLoader.loadClass(ClassLoader.java:252)
at java.lang.ClassLoader.loadClassInternal(ClassLoader.java:320)
at com.itextpdf.text.pdf.PdfEncryption.(PdfEncryption.java:147)
at com.itextpdf.text.pdf.PdfWriter.setEncryption(PdfWriter.java:2041)
at net.viralpatel.pdf.main(GeneratePDF.java:35)
Hi Venkata, Check the JDK version you using to compile and run this project. I have used JDK 1.6. The bouncycastle library that I used is also for JDK1.6. Download appropriate version and rerun the project.
Delete apacheds-all-1.5.4.jar irrespective of jdk version. I did it using jdk 1.7
Hello
You mention in your article that “Although not free, iText is the de-fecto Java library for working with PDF files”. Our company Qoppa Software has been focusing on Java libraries to work with PDF documents for more than 10 years. Our suite of libraries supports most PDF functions including manipulating (pretty much anything), viewing, printing, annotating. For what you’re doing our library jPDFSecure is the library you should be looking at. It can set passwords and permissions on PDF documents. Price starts at $350 for a dual core server. If you have any questions with our libraries, we’re here to answer at [email protected]. Also all our libraries are compatible with JDK 1.4 and higher.
Hi
I tried that above code its showing
But I used bcmail for JDk 1.7 jar file only
Hi, This error comes when classes belonging to the same package are loaded from different JAR files, and those JAR files have signatures signed with different certificates. Check if you have only one version of org.bouncycastle.asn1.ASN1ObjectIdentifier being loaded by checking all JAR files and see if more than one Jar have this class.
remove apache all library and it should work
Add this jar file to your code…(bcprov-jdk16-140.jar)
Hi,
I am also getting this error. I have checked my jdk version also .It is jdk1.6.24
Also i am using this itextpdf jar only. Kindly tell the way forward
Hoping that you will reply soon on this
I have also face the java.lang.SecurityException: class “org.bouncycastle.asn1.ASN1ObjectIdentifier”‘s signer information does not match signer information of other classes in the same package.
I am able to solve it.
Do not include apacheds-all-1.5.4.jar in your workspace. The code provided by VP contain the above mentioned jar. That is not required.
Jar required are –
* itextpdf-5.2.1.jar
* bcmail-jdk16-1.46.jar
* bcprov-jdk16-1.46.jar
* bctsp-jdk16-1.46.jar
Yes its working.After removing the apacheds-all-1.5.4.jar .Thank you
Thnx a lot ……
Dosen’t work for me. When I use these dependencies:
itextpdf-5.2.1.jar
bcmail-jdk16-1.46.jar
bcprov-jdk16-1.46.jar
bctsp-jdk16-1.46.jar
apacheds-all-1.5.4.jar (same without it)
I get this error: com.itextpdf.text.exceptions.InvalidPdfException: class “org.bouncycastle.asn1.ASN1ObjectIdentifier”‘s signer information does not match signer information of other classes in the same package
Have been trying so many different versions of Bouncy Castle now without any luck. What I’m I doing wrong? Btw. I use jdk 6u27
Delete apacheds-all-1.5.4.jar
Hi Viral Patel ,
This is Excellent and simple one . It helped me lot . But i need to deny the user from copy, save , print . what Constant i need to pass to the encrypt Function .
Thanks in advance.
Exception in thread “main” java.lang.NoClassDefFoundError: org/bouncycastle/asn1/DEREncodable
at com.lowagie.text.pdf.PdfEncryption.(Unknown Source)
at com.lowagie.text.pdf.PdfWriter.setEncryption(Unknown Source)
at com.prs.memberonline.ui.CreatePDFDataWithPassword.print(CreatePDFDataWithPassword.java:99)
at com.prs.memberonline.ui.CreatePDFDataWithPassword.main(CreatePDFDataWithPassword.java:469)
i got the above error while running the class. i downloaded jdk1.4 compatable jars and run it but still it is giving error
Please check this SO thread: http://stackoverflow.com/questions/10391271/itext-bouncycastle-classnotfound-org-bouncycastle-asn1-derencodable-and-org-boun.
Hi Viral Patel ,
This is Excellent and very nice post.
File is generated plse check it java.io.FileOutputStream@1decdec
page number is :0
after file is generated com.itextpdf.text.pdf.PdfWriter@37504d
java.lang.SecurityException: class “org.bouncycastle.asn1.ASN1ObjectIdentifier”‘s signer information does not match signer information of other classes in the same package
at java.lang.ClassLoader.checkCerts(Unknown Source)
at java.lang.ClassLoader.preDefineClass(Unknown Source)
at java.lang.ClassLoader.defineClass(Unknown Source)
at java.security.SecureClassLoader.defineClass(Unknown Source)
at java.net.URLClassLoader.defineClass(Unknown Source)
at java.net.URLClassLoader.access$100(Unknown Source)
at java.net.URLClassLoader$1.run(Unknown Source)
at java.net.URLClassLoader$1.run(Unknown Source)
at java.security.AccessController.doPrivileged(Native Method)
at java.net.URLClassLoader.findClass(Unknown Source)
at java.lang.ClassLoader.loadClass(Unknown Source)
at sun.misc.Launcher$AppClassLoader.loadClass(Unknown Source)
at java.lang.ClassLoader.loadClass(Unknown Source)
at com.itextpdf.text.pdf.PdfEncryption.(PdfEncryption.java:147)
at com.itextpdf.text.pdf.PdfWriter.setEncryption(PdfWriter.java:2041)
at com.nantech.PdfPassword.GeneratePDF.main(GeneratePDF.java:40)
Hi Virat,
Could you please let me know how to apply password for a Zip file using Java.
Thanks in adv!!
I just added the
private static String USER_PASS = “Hello123”;
private static String OWNER_PASS = “Owner123”; and
writer.setEncryption(USER_PASS.getBytes(), OWNER_PASS.getBytes(),
PdfWriter.ALLOW_PRINTING, PdfWriter.ENCRYPTION_AES_128);
in already existing program of generating pdf but i am getting an error PdfWriter.ENCRYPTION_AES_128 cannot be resolved..wat to do..?
I created pdf file successfully but Its Not opening with the password Hello123 and also Owner123 also Please give me some suggestion.
Hi VP
I did all the steps which you have mentioned, but i am getting error
Exception in thread “main” java.lang.NoClassDefFoundError: org/bouncycastle/asn1/ASN1Primitive
at com.itextpdf.text.pdf.PdfEncryption.(PdfEncryption.java:149)
at com.itextpdf.text.pdf.PdfWriter.setEncryption(PdfWriter.java:2119)
at com.EncryptPdf.GenerateEncrptPdf.main(GenerateEncrptPdf.java:24)
Caused by: java.lang.ClassNotFoundException: org.bouncycastle.asn1.ASN1Primitive
at java.net.URLClassLoader$1.run(Unknown Source)
at java.net.URLClassLoader$1.run(Unknown Source)
at java.security.AccessController.doPrivileged(Native Method)
at java.net.URLClassLoader.findClass(Unknown Source)
at java.lang.ClassLoader.loadClass(Unknown Source)
at sun.misc.Launcher$AppClassLoader.loadClass(Unknown Source)
at java.lang.ClassLoader.loadClass(Unknown Source)
… 3 more
I am using jdk1.7 PDF file is generating but i cant access the file. Its coming like
It is either not supported file or because the file has been damaged..
what to do..?
Is there a way to customize or change the message that gets displayed in the document open Password dialog box while trying to open a password protected PDF file.
Default message – “filename.pdf is protected. please enter a Document Open Password.”
1. Save GeneratePDF.java file in :\Java\jdk1.6.0_05\bin
2. copy lib File (Java_iText_PDF_Password\Java_iText_PDF_Password\lib to :\Java\jdk1.6.0_05\jre\lib\ext
3. Run Compile and run java program “javac GeneratePDF.java ” then java GeneratePDF.java
4. Same drive created open Test.pdf file and enter Hello123 password
5. Done
http://t.co/sFqvP1wsH4
Here is another way for that. Set restrictions for opening, copying, editing, and printing to make sure your files are safe even if they fall into the wrong hands.
I have facing problem while running above same code , I am use Java 1.7 .
java.lang.SecurityException: class “org.bouncycastle.asn1.ASN1ObjectIdentifier”‘s signer information does not match signer information of other classes in the same package
at java.lang.ClassLoader.checkCerts(ClassLoader.java:952)
at java.lang.ClassLoader.preDefineClass(ClassLoader.java:666)
at java.lang.ClassLoader.defineClass(ClassLoader.java:794)
at java.security.SecureClassLoader.defineClass(SecureClassLoader.java:142)
at java.net.URLClassLoader.defineClass(URLClassLoader.java:449)
at java.net.URLClassLoader.access$100(URLClassLoader.java:71)
at java.net.URLClassLoader$1.run(URLClassLoader.java:361)
at java.net.URLClassLoader$1.run(URLClassLoader.java:355)
at java.security.AccessController.doPrivileged(Native Method)
at java.net.URLClassLoader.findClass(URLClassLoader.java:354)
at java.lang.ClassLoader.loadClass(ClassLoader.java:425)
at sun.misc.Launcher$AppClassLoader.loadClass(Launcher.java:308)
at java.lang.ClassLoader.loadClass(ClassLoader.java:358)
at com.itextpdf.text.pdf.PdfEncryption.(PdfEncryption.java:147)
at com.itextpdf.text.pdf.PdfWriter.setEncryption(PdfWriter.java:2041)
at sample.PdfGeneration.main(PdfGeneration.java:20)
i am using following jar files.
bctest-jdk15on-154.jar
bcpg-jdk15on-154.jar
bcmail-jdk15on-154.jar
bcpkix-jdk15on-154.jar
bcprov-ext-jdk15on-154.jar
bcprov-jdk15on-154.jar
bcmail-jdk15on-1.54-javadoc.jar
apacheds-all-1.5.4.jar
itextpdf-5.2.1.jar
anybody help me how to resolve the issue ?
Which version of iText supports AES 256 Encryption algorithm.
Pankaj, got any option for your post ?
Itext
Is there a way to customize or change the message that gets displayed in the document open Password dialog box while trying to open a password protected PDF file.
Default message – “filename.pdf is protected. please enter a Document Open Password.”