Following is an example of JavaScript where we will remove elements from an array with Index and Value. There are different methods in JavaScript that we can use to remove elements. We will use
To test the above code lets define a simple array and remove one of the element.
splice()
method.Remove Array element by Index
First we will see an example were we will remove array element by its index. For this we can define a generic function removeByIndex().function removeByIndex(arr, index) {
arr.splice(index, 1);
}
Code language: JavaScript (javascript)
In above code we used javascript splice()
method to remove element at an index. splice() method takes two argument. First is the index at which you want to remove element and second is number of elements to be removed. In our case it is 1. 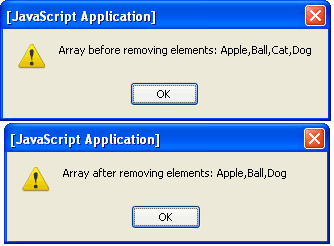
test = new Array();
test[0] = 'Apple';
test[1] = 'Ball';
test[2] = 'Cat';
test[3] = 'Dog';
alert("Array before removing elements: "+test);
removeByIndex(test, 2);
alert("Array after removing elements: "+test);
Code language: JavaScript (javascript)
Also you may want to define removeByIndex method with an array so that you call it directly. For example, in following example, we have define an array test and its method removeByIndex().test = new Array();
//define removeByIndex method as part of the array
Array.prototype.removeByIndex = function(index) {
this.splice(index, 1);
}
test[0] = 'Apple';
test[1] = 'Ball';
test[2] = 'Cat';
test[3] = 'Dog';
alert("Array before removing elements: "+test);
test.removeByIndex(2);
alert("Array after removing elements: "+test);
Code language: JavaScript (javascript)
Remove Array element by Value
You may want to remove array element by its value and not index. This can be easily done by iterating through it and comparing the values, if a match is found we can remove that element bysplice()
method.function removeByValue(arr, val) {
for(var i=0; i<arr.length; i++) {
if(arr[i] == val) {
arr.splice(i, 1);
break;
}
}
}
Code language: JavaScript (javascript)
The above code can be written as part of array also:test = new Array();
Array.prototype.removeByValue = function(val) {
for(var i=0; i<this.length; i++) {
if(this[i] == val) {
this.splice(i, 1);
break;
}
}
}
test[0] = 'Apple';
test[1] = 'Ball';
test[2] = 'Cat';
test[3] = 'Dog';
alert("Array before removing elements: "+test);
test.removeByValue('Cat');
alert("Array after removing elements: "+test);
Code language: JavaScript (javascript)
Enjoy…
very cool & good tip, thank you very much for sharing.
Can I share this post on my JavaScript library?
Awaiting your response. Thank
thanks, very helpful, this is what I was looking for.
Spot on – thanks
Thanks Steve :-)
Usually, I don’t leave comments, but I have to say this was exactly what I was looking for and it’s very well explained. Thank you VERY much!
Thanks very much for the script, i was looking for this to use in my site. removeByValue make my day…
Thanks Viral, Good explanation
Thanks a lot for a wonderful explanation
It will only remove first occurrence!
Thanks for sharing the information, nice it save’s my time
Hey Viral,
Thanks for sharing this a great post on removing JavaScript array elements!