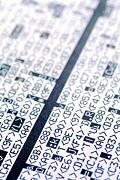
If you want to work with Comma-separated Files (CSV) in Java, here’s a quick API for you. As Java doesn’t support parsing of CSV files natively, we have to rely on third party library. Opencsv is one of the best library available for this purpose. It’s open source and is shipped with Apache 2.0 licence which makes it possible for commercial use. Let’s us see different APIs to parse CSV file. Before that we will need certain tools for this example:
Tools & Technologies
- Java JDK 1.5 or above
- OpenCSV library v1.8 or above (download)
- Eclipse 3.2 above (optional)
Let’s get started.
1. Reading CSV file in Java
We will use following CSV sample file for this example: File: sample.csv
COUNTRY,CAPITAL,POPULATION
India,New Delhi, 1.21B
People's republic of China,Beijing, 1.34B
United States,Washington D.C., 0.31B
Code language: PHP (php)
Read CSV file line by line:
String csvFilename = "C:\\sample.csv";
CSVReader csvReader = new CSVReader(new FileReader(csvFilename));
String[] row = null;
while((row = csvReader.readNext()) != null) {
System.out.println(row[0]
+ " # " + row[1]
+ " # " + row[2]);
}
//...
csvReader.close();
Code language: Java (java)
In above code snippet, we use readNext()
method of CSVReader
class to read CSV file line by line. It returns a String array for each value in row. It is also possible to read full CSV file once. The readAll()
method of CSVReader class comes handy for this.
String[] row = null;
String csvFilename = "C:\\work\\sample.csv";
CSVReader csvReader = new CSVReader(new FileReader(csvFilename));
List content = csvReader.readAll();
for (Object object : content) {
row = (String[]) object;
System.out.println(row[0]
+ " # " + row[1]
+ " # " + row[2]);
}
//...
csvReader.close();
Code language: Java (java)
The readAll()
method returns a List
of String[]
for given CSV file. Both of the above code snippet prints output: Output
COUNTRY # CAPITAL # POPULATION
India # New Delhi # 1.21B
People's republic of China # Beijing # 1.34B
United States # Washington D.C. # 0.31B
Code language: PHP (php)
Use different separator and quote characters If you want to parse a file with other delimiter like semicolon (;) or hash (#), you can do so by calling a different constructor of CSVReader class:
CSVReader reader = new CSVReader(new FileReader(file), ';')
//or
CSVReader reader = new CSVReader(new FileReader(file), '#')
Code language: Java (java)
Also if your CSV file’s value is quoted with single quote (‘) instead of default double quote (“), then you can specify it in constructor:
CSVReader reader = new CSVReader(new FileReader(file), ',', '\'')
Code language: Java (java)
Also it is possible to skip certain lines from the top of CSV while parsing. You can provide how many lines to skip in CSVReader’s constructor. For example the below reader will skip 5 lines from top of CSV and starts processing at line 6.
CSVReader reader = new CSVReader(new FileReader(file), ',', '\'', 5);
Code language: Java (java)
2. Writing CSV file in Java
Creating a CSV file is as simple as reading one. All you have to do is it create the data list and write using CSVWriter
class. Below is the code snippet where we write one line in CSV file.
String csv = "C:\\output.csv";
CSVWriter writer = new CSVWriter(new FileWriter(csv));
String [] country = "India#China#United States".split("#");
writer.writeNext(country);
writer.close();
Code language: Java (java)
We created object of class CSVWriter
and called its writeNext()
method. The writeNext()
methods takes String []
as argument. You can also write a List of String[] to CSV directly. Following is code snippet for that.
String csv = "C:\\output2.csv";
CSVWriter writer = new CSVWriter(new FileWriter(csv));
List<String[]> data = new ArrayList<String[]>();
data.add(new String[] {"India", "New Delhi"});
data.add(new String[] {"United States", "Washington D.C"});
data.add(new String[] {"Germany", "Berlin"});
writer.writeAll(data);
writer.close();
Code language: Java (java)
We used writeAll()
method of class CSVWriter to write a List of String[] as CSV file.
3. Mapping CSV with Java beans
In above examples we saw how to parse CSV file and read the data in it. We retrieved the data as String array. Each record got mapped to String. It is possible to map the result to a Java bean object. For example we created a Java bean to store Country information. Country.java – The bean object to store Countries information.
package net.viralpatel.java;
public class Country {
private String countryName;
private String capital;
public String getCountryName() {
return countryName;
}
public void setCountryName(String countryName) {
this.countryName = countryName;
}
public String getCapital() {
return capital;
}
public void setCapital(String capital) {
this.capital = capital;
}
}
Code language: Java (java)
Now we can map this bean with Opencsv and read the CSV file. Check out below example:
ColumnPositionMappingStrategy strat = new ColumnPositionMappingStrategy();
strat.setType(Country.class);
String[] columns = new String[] {"countryName", "capital"}; // the fields to bind do in your JavaBean
strat.setColumnMapping(columns);
CsvToBean csv = new CsvToBean();
String csvFilename = "C:\\sample.csv";
CSVReader csvReader = new CSVReader(new FileReader(csvFilename));
List list = csv.parse(strat, csvReader);
for (Object object : list) {
Country country = (Country) object;
System.out.println(country.getCapital());
}
Code language: Java (java)
Check how we mapped Country
class using ColumnPositionMappingStrategy
. Also the method setColumnMapping
is used to map individual property of Java bean to the CSV position. In this example we map first CSV value to countryName
attribute and next to capital
.
4. Dumping SQL Table as CSV
OpenCSV also provides support to dump data from SQL table directly to CSV. For this we need ResultSet object. Following API can be used to write data to CSV from ResultSet.
Code language: Java (java)java.sql.ResultSet myResultSet = getResultSetFromSomewhere(); writer.writeAll(myResultSet, includeHeaders);
The writeAll(ResultSet, boolean)
method is utilized for this. The first argument is the ResultSet which you want to write to CSV file. And the second argument is boolean which represents whether you want to write header columns (table column names) to file or not.
Download Source Code
ReadWrite_CSV_Java_example.zip (356 KB)
Very helpful tutorials!! keep it up. Cheers
Tu yahan par bhi pohach gaya ;)
keep it up????????
Hi SIr,
I want to display one row data into another row with same values .
e.g. first row contains same value after reading program out file will be 2 rows same values plzz help me
Hi SIr,
I want to display one row data into another row with same values .
e.g. first row contains same value after reading program output file will be 2 rows same values
plzz help me..Thanks
hi..
I want to know about how to map csv file with database.my project on J2EE so please help me.how to view ,update ,upload,delete csv file .thank you.
Thank you for this post.
A question: how would you deal with values that contain commas? For example if there’s a company name Some Company, Inc.. The usual parsing will interpret the comma between company and Inc as separate values, and this throws everything off.
I have no control over the csv file — I need to work with it the way it is.
Thanks!
man after a long search I found you, and I hope you’ll relieve me of my frustration. Thing is I’ve .CSV files and .CSV data dumps from a website for which im an affiliate and when i’m trying to upload them to my wordpress website nothing is working!
I just want to upload my .CSV and make galleries on my webpage, pls help me out with this; could you suggest any csv plugin that works with wordpress? I failed with everything available. I’m clueless!
Thanks in advance
Hello Mr Patel
I have to prepare a java tool which can combine multiple csv files into a single excel sheet where each of these csv file will represent a single worksheet of the combined excel file . Can you please help me in getting a little insight on the logical approach which will be involved in developing the road map of this tool . you please help me at the earliest .
Regards
Prag Tyagi
thanks It helped.
JavaOne 2013 is apparently being held at Hyderabad this year as well (8-9 May) at the same place guys…. They have also started accepting registrations (check this: http://bit.ly/YMPeJ8 )
I am definitely going to attend the Java One again. Last time I had a chance to help people at NetBeans Booth, thanks to Java One Team for that great favor. I know, Java and NetBeans community will definitely attend this prestigious nd great conference this year too…..
How to update CSV files using openCSV ??
Hi Viral,
Can you please tell me which is the best CSV reader provided by different opensource stuff.
ex. Apace,Sourceforge have their csvReader API.
In the same way,i need a CSVreader which can read and parse the file to a List of Records Objects.You can suggest any other also.
Please reply me asap.
Thanks in advance!!
When I am using the above code, it is not fetching the complete data, however its fetching data from in between.
Any pointers…
You may want to look at Ostermiller CSV parser (http://ostermiller.org/utils/CSV.html)
I have been using it for the past 8+ years and it is too good.
Hi Viral,
I am facing a strange issue while reading the csv rows, the values are appended with special characters at the end of the String. I am reading and coverting to Bean using CsvToBean like this
CSVReader csvReader = new CSVReader(new FileReader(chargebackFileName),
‘,’,
‘\”‘,
‘\\’,
2, false, true);
Can you help with what can be the issue.
Thanks
Very Nyc Tutorials…
Good tutuorial! Very helpful
Very Hepful.. thanks a lot!
Cool!!
Hi Viral, Take a look at bindy for .csv files. It supports annotations as well. Great work !
how to validate date format in that .i dont know please tell me its urgent to me.
vary useful one
Thanks a lot. Very Use full guide.
can openCSV be used in mutithreaded environment.
hi dude,
this information is very useful to me… keep it up and you have any idea about file API’s other than JAVA FILE API’s pls listing out in the site….
ok,not bad
Hi Viral Patel, thank you so much. The article helped me to achieve a task. Others articles in others pages only gave me a headache. This is very helpful.
Hello! Sir
can you help me to write a code, in which i have to read a csv file ,
“Sumit”,”B.H Singh”,”1500″,”45″,”no”,”53″
“Pragya Rani”,”Romesh Kumar”,”1600″,”47″,”no”,”56″
“Ravina Singh”,”Jagjit Singh”,”1580″,”48″,”yes”,”76″
“Rajit Sharma”,”Hardik Sharma”,”1630″,”50″,”no”,”67″
“Shilpa Sharma”,”B.K Sharma”,”1590″,”42″,”no”,”67″
“Tanvi Verma”,”Suresh Singh”,”1620″,”38″,”yes”,”78″
“Ritu Sharma”,”Naresh Sharma”,”1530″,”35″,”yes”,”34″
“Anmol Garg”,”Atul Garg”,”1570″,”40″,”yes”,”62″
//… skip, too large
then sort it according to last column,
then write it to another text file in sorted way.
private static void readLineByLineExample() throws IOException
{
BufferedReader CSVFile =
new BufferedReader(new FileReader(“C:\\Users\\Desktop\\std_db.sql”));
String dataRow = CSVFile.readLine(); // Read first line.
// The while checks to see if the data is null. If
// it is, we’ve hit the end of the file. If not,
// process the data.
while (dataRow != null)
{
String[] dataArray = dataRow.split(“,”);
for (String item:dataArray)
{
System.out.print(item + “\t”);
}
System.out.println(); // Print the data line.
dataRow = CSVFile.readLine(); // Read next line of data.
}
// Close the file once all data has been read.
CSVFile.close();
// End the printout with a blank line.
System.out.println();
}
hi this is the code which i am runnig for cvs file reader..but its not working :( what is wrong?
import java.io.*;
import java.util.Arrays;
public class CSVRead {
public static void main(String[] arg) throws Exception {
BufferedReader CSVFile = new BufferedReader(new FileReader(
“D:\\aaTamilpersonnel\\12 may 2014\\part-00000(1)”));
String dataRow = CSVFile.readLine();
while (dataRow != null) {
String[] dataArray = dataRow.split(“\t”);
for (String item : dataArray) {
System.out.print(item + “\t”);
}
System.out.println(); // Print the data line.
dataRow = CSVFile.readLine(); // Read next line of data.
}
CSVFile.close();
// End the printout with a blank line.
System.out.println();
}
}
This code will work . Make sure that you are using correct delimiter.
Hi,its very helpful but is it possible to update particular cell value using open csv?keeping all row elements same ?
Good time saving article
can you just give an idea how to ceate xml from .csv file.
u cant create xml from csv cz its impossible… :p
Type mismatch: cannot convert from List to List
Syntax error on token “add”, = expected after this token
Syntax error on token “data”, VariableDeclaratorId expected after this token
Syntax error on token “data”, VariableDeclaratorId expected after this token
Syntax error on token “data”, VariableDeclaratorId expected after this token
Syntax error on token “close”, Identifier expected after this token
if my csv file is like this
ID,NAME
1,xyz
2,pqr
,
3,abc
————————END
How i can get exact row where data is present??? that is 1,2,3 i must get at the end. Can you help me for this?
Great tutorial, Thank you. However, I was wondering why they would have an overloaded constructor using different delimiter for CSVReader?. The class name implies that it is going to read Comma Separated Values, where Comma is the delimiter. Maybe DSVReader would have been a better name.
Thank you Viral, this was very helpful. Reduced my lines of code significantly. Keep up the good job.
Thanks for this post.. its very helpful…!
nice work…
Thanks for the article, and I’m sharing one more open-source library for reading/writing/mapping CSV data. Since I used this library in my project, I found out it powerful and flexiable especially parsing big CSV data (such as 1GB+ file or complex processing logic).
Here are the code snippts programming the functionalities included in this article:
1. Reading CSV file in Java:
2. Writing CSV file in Java:
3. Mapping CSV with Java beans
Hello, what if I would like to update/delete a row?
Have I to delete the old line and replace it with the new one?
thanks!
i have csv file that contain
XYZ,nirav,{“longo”:25,”lat”:35.2},72.32
i need to Split when reading csv file as follwing..
XYZ
nirav
{“longo”:25,”lat”:35.2}
72.32
what is way to do this..
i m using –> line.split(‘,”); so i get
XYZ
nirav
{“longo”:25
“lat”:35.2}
72.32 but i want as following
i need to Split when reading csv file as follwing..
XYZ
nirav
{“longo”:25,”lat”:35.2}
72.32
CSVReader reader = new CSVReader(new FileReader(file), ‘,’, ‘\”, 5);
this ‘\” remove \ from the value also
any idea about that
Hello, i am unable to download source code, any help ?
Hey mate thanks for this, how would I go about making a loop to add data from a textField or something?
how to read csv file and convert html format in java ?
Anyone can please tell me how to add Opencsv folder with eclipse and we add or import the library file to java class file.Please help me .I don’t know how to attach opencsv with eclipse java class file.It would help me alot.Please Respond.
Thank you