HTML5 Datalist is a new html tag shipped with HTML5 specification. HTML5 Datalist can be used to create a simple poor man’s Autocomplete feature for a webpage.
In this tutorial we will go through the basics of HTML5 Datalist tag and check some quick examples of autocomplete.
Introduction to HTML5 Datalist tag
As part of HTML5 specification a new tag <datalist>
has been introduced. Using this tag, we can define a list of data which then can be used as list for an input box. We can create a simple Autocomplete feature using this tag.
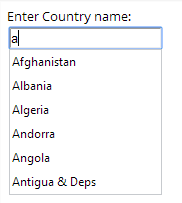
Consider a textbox to take country name input from user.
Syntax:
<input type="text" list="countries" name="mycountry" />
Code language: HTML, XML (xml)
See how we used attribute list and pass a list of countries called ‘countries’. We can define this list of countries using datalist tag.
<datalist id="countries">
<option value="India">India</option>
<option value="United States">United States</option>
<option value="United Kingdom">United Kingdom</option>
<option value="Germany">Germany</option>
<option value="France">France</option>
</datalist>
Code language: HTML, XML (xml)
So we created a list of countries using <datalist>
tag. The id of datalist tag must match with the list attribute of the input box.
Demo
Below is the demo. Enter some text in the Country textbox and see how an autocomplete list appears below it.
Browser Compatibility
Not every browser out there supports html5 datalist for now. So far IE 10 and above, Firefox, Chrome and Opera 19 above supports html5 datalist tag. Safari doesn’t support datalist yet :(
Chrome | Firefox (Gecko) | Internet Explorer | Opera | Safari |
---|---|---|---|---|
31 | 26.0 | 10.0 | 19.0 | – |
This might change in future. Check the latest compatibility matrix for HTML5 Datalist here http://caniuse.com/#search=datalist.
Backward Compatibility for HTML5 Datalist tag
As seen in above compatibility table, not all browser supports html5 datalist tag. If you are using datalist, the older browser will simply ignores the datalist tag and renders rest of the webpage. The textbox which is linked to datalist will remain just a textbox. No autocomplete will be added. Internet explorer 9 and below has unique way of parsing datalist. If you try to check above example in IE 9 or below you will see all the option values are simply printed on the page. To avoid this we can wrap the options in datalist tag with a select tag. For example:
<datalist id="countries">
<select>
<option value="India">India</option>
<option value="United States">United States</option>
<option value="United Kingdom">United Kingdom</option>
<option value="Germany">Germany</option>
<option value="France">France</option>
</select>
</datalist>
Code language: HTML, XML (xml)
This can give user option to select from the list if autocomplete is not available. Check below screenshot.
Here we simple let user enter the country name or they can select from the country list box. This way users have an option to select from the list if they want to.
This can be simply achieve by adding a select tag inside datalist and make it as if it’s a select box.
Demo:
Here we simply wrapped all options within select tag. The name of select tag must be same as that of textbox. So when the form is submitted the appropriate value is passed to server-side code.
This is crud way of doing backward compatibility. If you don’t want to add more javascripts to your page, you can use this simple technique.
There is a better way of adding datalist compatibility to browsers that aren’t yet supporting this feature. We call it Polyfills. It is a way to fallback functionality to older browsers.
Chris Coyier has written a nice library that polyfills the datalist capabilities into older/non-supportive browsers.
All we need to do is to check if our browser supports datalist of not. If not then simply includes the polyfill js library. Check out below example.
We use Modernizr to identify if browser supports html5 datalist, if not then we simply includes the polyfill javascript for datalist by Chris Coyier.
<!DOCTYPE html>
<html>
<head>
<script src="http://cdnjs.cloudflare.com/ajax/libs/modernizr/2.7.1/modernizr.min.js"></script>
<script>
// Safari reports success of list attribute, so doing ghetto detection instead
yepnope({
test : (!Modernizr.input.list || (parseInt($.browser.version) > 400)),
yep : [
'https://raw2.github.com/CSS-Tricks/Relevant-Dropdowns/master/js/jquery.relevant-dropdown.js',
'https://raw2.github.com/CSS-Tricks/Relevant-Dropdowns/master/js/load-fallbacks.js'
]
});
</script>
</head>
<body>
<input type="text" list="countries" name="mycountry" />
<datalist id="countries">
<option value="India">India</option>
<option value="United States">United States</option>
<option value="United Kingdom">United Kingdom</option>
<option value="Germany">Germany</option>
<option value="France">France</option>
</datalist>
</body>
</html>
Code language: HTML, XML (xml)
Will this datalist any way collide with the autocomplete? If there is India in the Autocomplete and in the datalist, what happens then?
Good point Kaushik. Browsers internal autocomplete will definitely collide with the datalist. In such case we can disable browser’s internal autocomplete using
autocomplete="off"
attribute. For example:caution with the autocomplete=off the browser will probably search for the “off” list…
if it dosn’t exist, don’t worry that’s like
who will show the control buttons
tip: removeAttribute in javascript or not…
Thanks for sharing such a detailed datalist explanation!!! Nice article!!
awesome post , i never know this before .
I was doing in angular or using jquery .This is very simple .
Thanks for sharing
Nice post! With Google Chrome, at least with version 34, the datalist gets cut off if it’s too long, and a user can’t scroll up or down to see the rest of the items, unlike with Firefox and IE 11. Is there a way to have Chrome let users see all the options in a long list? Here’s an example of a full country list: http://jsfiddle.net/amGY9/15/
Thanks!
Hi,
i am using datalist in my web application. It works find in IE 10 in my local which is in windows environment. But the same web app when run from a server which is running in Linux shows the list of data out of the text box. have tried all the above mentioned fixes but could not fix the problem. can you expalin me why and suggest a solution to fix the Issue.
Regards,
Joseph
nice tutor,
thanks
nice, no need ajax as the list can be there
Can we keep limit on no of items displayed ?
i want user must be select value in datalist value only then what i will do????
How can we add the scroll while showing datalist?
this seems not working on mine.. ):
How can we use autocomplete with HTML5 & jquery?..here in the demo we are giving the list values in the option tags..but how can we fetch with using javascript?
Can ajax be combined with datalist?..can u please provide with a sample demo?
thank you, works
Nice Article. HTML 5 has proven to be a boon for Web Development. I really like the HTML 5 API stuff and Offline content features.
can data list support scroll?