Welcome to Hibernate Tutorial Series. In previous tutorials we saw how to implement Inheritance in Hibernate: One Table Per Hierarchy.
Today we will see how to implement Hibernate Inheritance: One Table per Subclass scheme.
Introduction to Inheritance in Hibernate
Java is an object oriented language. It is possible to implement Inheritance in Java. Inheritance is one of the most visible facets of Object-relational mismatch. Object oriented systems can model both “is a” and “has a” relationship. Relational model supports only “has a” relationship between two entities. Hibernate can help you map such Objects with relational tables. But you need to choose certain mapping strategy based on your needs.
One Table Per Subclass example
Suppose we have a class Person with subclass Employee and Owner. Following the class diagram and relationship of these classes.
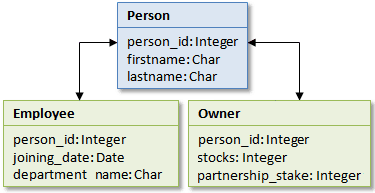
In One Table per Subclass scheme, each class persist the data in its own separate table. Thus we have 3 tables; PERSON, EMPLOYEE and OWNER to persist the class data. Note that a foreign key relationship exists between the subclass tables and super class table. Thus the common data is stored in PERSON table and subclass specific fields are stored in EMPLOYEE and OWNER tables.
Following are the advantages and disadvantages of One Table per Subclass scheme.
Advantage
- Using this hierarchy, does not require complex changes to the database schema when a single parent class is modified.
- It works well with shallow hierarchy.
Disadvantage
- As the hierarchy grows, it may result in poor performance.
- The number of joins required to construct a subclass also grows.
Create Database Table to persist Subclass
CREATE TABLE `person` (
`person_id` BIGINT(20) NOT NULL AUTO_INCREMENT,
`firstname` VARCHAR(50) NOT NULL DEFAULT '0',
`lastname` VARCHAR(50) NOT NULL DEFAULT '0',
PRIMARY KEY (`person_id`)
)
CREATE TABLE `employee` (
`person_id` BIGINT(10) NOT NULL,
`joining_date` DATE NULL DEFAULT NULL,
`department_name` VARCHAR(50) NULL DEFAULT NULL,
PRIMARY KEY (`person_id`),
CONSTRAINT `FK_PERSON` FOREIGN KEY (`person_id`) REFERENCES `person` (`person_id`)
)
CREATE TABLE `owner` (
`person_id` BIGINT(20) NOT NULL DEFAULT '0',
`stocks` BIGINT(11) NULL DEFAULT NULL,
`partnership_stake` BIGINT(11) NULL DEFAULT NULL,
PRIMARY KEY (`person_id`),
CONSTRAINT `FK_PERSON2` FOREIGN KEY (`person_id`) REFERENCES `person` (`person_id`)
)
Code language: SQL (Structured Query Language) (sql)
Hibernate Inheritance: XML Mapping
Following is the example where we map Person, Employee and Owner entity classes using XML mapping.
File: Person.java
package net.viralpatel.hibernate;
public class Person {
private Long personId;
private String firstname;
private String lastname;
// Constructors and Getter/Setter methods,
}
Code language: Java (java)
File: Employee.java
package net.viralpatel.hibernate;
import java.util.Date;
public class Employee extends Person {
private Date joiningDate;
private String departmentName;
// Constructors and Getter/Setter methods,
}
Code language: Java (java)
File: Owner.java
package net.viralpatel.hibernate;
public class Owner extends Person {
private Long stocks;
private Long partnershipStake;
// Constructors and Getter/Setter methods,
}
Code language: Java (java)
File: Person.hbm.xml
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE hibernate-mapping PUBLIC
"-//Hibernate/Hibernate Mapping DTD 3.0//EN"
"http://hibernate.sourceforge.net/hibernate-mapping-3.0.dtd">
<hibernate-mapping package="net.viralpatel.hibernate">
<class name="Person" table="PERSON">
<id name="personId" column="PERSON_ID">
<generator class="native" />
</id>
<property name="firstname" />
<property name="lastname" column="lastname" />
<joined-subclass name="Employee" extends="Person">
<key column="person_id" />
<property name="departmentName" column="department_name" />
<property name="joiningDate" type="date" column="joining_date" />
</joined-subclass>
<joined-subclass name="Owner" extends="Person">
<key column="person_id" />
<property name="stocks" column="stocks" />
<property name="partnershipStake" column="partnership_stake" />
</joined-subclass>
</class>
</hibernate-mapping>
Code language: Java (java)
Note that we have defined only one hibernate mapping (hbm) file Person.hbm.xml
. Both Person
and Employee
model class are defined within one hbm file.
<discriminator> tag is used to define the discriminator column.
<subclass> tag is used to map the subclass Employee. Note that we have not used the usual <class>
tag to map Employee as it falls below in the hierarchy tree.
The discriminator-value for Person
is defined as “P” and that for Employee
is defined “E”, Thus, when Hibernate will persist the data for person or employee it will accordingly populate this value.
Hibernate Inheritance: Annotation Mapping
Following is the example where we map Employee and Person entity classes using JPA Annotations.
File: Person.java
package net.viralpatel.hibernate;
import javax.persistence.Column;
import javax.persistence.Entity;
import javax.persistence.GeneratedValue;
import javax.persistence.Id;
import javax.persistence.Inheritance;
import javax.persistence.InheritanceType;
import javax.persistence.Table;
@Entity
@Table(name = "PERSON")
@Inheritance(strategy=InheritanceType.JOINED)
public class Person {
@Id
@GeneratedValue
@Column(name = "PERSON_ID")
private Long personId;
@Column(name = "FIRSTNAME")
private String firstname;
@Column(name = "LASTNAME")
private String lastname;
public Person() {
}
public Person(String firstname, String lastname) {
this.firstname = firstname;
this.lastname = lastname;
}
// Getter and Setter methods,
}
Code language: Java (java)
The Person
class is the root of hierarchy. Hence we have used some annotations to make it as the root.
@Inheritance
– Defines the inheritance strategy to be used for an entity class hierarchy. It is specified on the entity class that is the root of the entity class hierarchy.
@InheritanceType
– Defines inheritance strategy options. JOINED is a strategy in which fields that are specific to a subclass are mapped to a separate table than the fields that are common to the parent class, and a join is performed to instantiate the subclass. Thus fields of Employee (joining_date, department) and Owner (stocks etc) are mapped to their respective tables.
File: Employee.java
package net.viralpatel.hibernate;
import java.util.Date;
import javax.persistence.Column;
import javax.persistence.Entity;
import javax.persistence.PrimaryKeyJoinColumn;
import javax.persistence.Table;
@Entity
@Table(name="EMPLOYEE")
@PrimaryKeyJoinColumn(name="PERSON_ID")
public class Employee extends Person {
@Column(name="joining_date")
private Date joiningDate;
@Column(name="department_name")
private String departmentName;
public Employee() {
}
public Employee(String firstname, String lastname, String departmentName, Date joiningDate) {
super(firstname, lastname);
this.departmentName = departmentName;
this.joiningDate = joiningDate;
}
// Getter and Setter methods,
}
Code language: Java (java)
File: Owner.java
package net.viralpatel.hibernate;
import javax.persistence.Column;
import javax.persistence.Entity;
import javax.persistence.PrimaryKeyJoinColumn;
import javax.persistence.Table;
@Entity
@Table(name="OWNER")
@PrimaryKeyJoinColumn(name="PERSON_ID")
public class Owner extends Person {
@Column(name="stocks")
private Long stocks;
@Column(name="partnership_stake")
private Long partnershipStake;
public Owner() {
}
public Owner(String firstname, String lastname, Long stocks, Long partnershipStake) {
super(firstname, lastname);
this.stocks = stocks;
this.partnershipStake = partnershipStake;
}
// Getter and Setter methods,
}
Code language: Java (java)
Both Employee and Owner classes are child of Person class. Thus while specifying the mappings, we used @PrimaryKeyJoinColumn
to map it to parent table.
@PrimaryKeyJoinColumn
– This annotation specifies a primary key column that is used as a foreign key to join to another table.
It is used to join the primary table of an entity subclass in the JOINED mapping strategy to the primary table of its superclass; it is used within a SecondaryTable annotation to join a secondary table to a primary table; and it may be used in a OneToOne mapping in which the primary key of the referencing entity is used as a foreign key to the referenced entity.
If no PrimaryKeyJoinColumn annotation is specified for a subclass in the JOINED mapping strategy, the foreign key columns are assumed to have the same names as the primary key columns of the primary table of the superclass
Main class
package net.viralpatel.hibernate;
import java.util.Date;
import org.hibernate.Session;
import org.hibernate.SessionFactory;
public class Main {
public static void main(String[] args) {
SessionFactory sf = HibernateUtil.getSessionFactory();
Session session = sf.openSession();
session.beginTransaction();
Person person = new Person("Steve", "Balmer");
session.save(person);
Employee employee = new Employee("James", "Gosling", "Marketing", new Date());
session.save(employee);
Owner owner = new Owner("Bill", "Gates", 300L, 20L);
session.save(owner);
session.getTransaction().commit();
session.close();
}
}
Code language: Java (java)
The Main class is used to persist Person
, Employee
and Owner
object instances. Note that these classes are persisted in different tables.
Output
Hibernate: insert into PERSON (firstname, lastname) values (?, ?)
Hibernate: insert into PERSON (firstname, lastname) values (?, ?)
Hibernate: insert into Employee (department_name, joining_date, person_id) values (?, ?, ?)
Hibernate: insert into PERSON (firstname, lastname) values (?, ?)
Hibernate: insert into Owner (stocks, partnership_stake, person_id) values (?, ?, ?)
Code language: SQL (Structured Query Language) (sql)
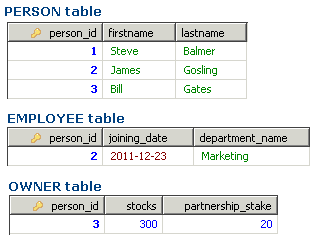
Download Source Code
Hibernate-inheritance-table-per-subclass_xml.zip (9 KB)
Hibernate-inheritance-table-per-subclass_annotation.zip (9 KB)
This is a very useful example and is presented clearly. Thanks you.
One correction – the ER diagram at the beginning does not indicate the correct columns for table “owner”. It indicates the same columns as for the parent table “person”.
@Gerry – Oops, my mistake :) I will update the ER diagram. Thanks!
Hi,
I followed the same and i get below exception
Can you help ?
I got it. This issue is because i missed the extend method in the joined-subclass element. After adding it worked.
@Inheritance(strategy=InheritanceType.JOINED)
This type of inheritance can cause you problems because hibernate will join all tables in hierarchy. Oracle admin can kick your ass because of such queries. I’ve seen tens of tables joined resulting very large and slow queries in production environments.
Hi,
first of all, I have to thank you for Struts2 and Hibernate tutorials. They are really helpful for me.
I’m doing a simple project similar to Struts2 and Hibernate example tutorial, but using Person and Owner. What I want to do is to add a new owner from a jsp, as you do in Struts2 and Hibernate example tutorial. I’m having some problems accesing to firstname or lastname properties.
owner.jsp code:
The action is called perfectly but it gives me this error when I try to add a new owner:
Error setting expression ‘owner.firstname’ with value ‘[Ljava.lang.String;@1312237’
ognl.OgnlException: target is null for setProperty(null, “firstname”, [Ljava.lang.String;@1312237)
I don’t know what I’m doing wrong.
Thanks in advance,
Alicia.
I finally found the error, it was related to properties’ getters and setters.
Nice document for beginners,who are learning SPRING and Hibernate.
Thanks,
Jyoti
hi,
Thanks for the wonderfully written hibernate tutorials.
Also wanted to let u know in one table per subclass hierarchy tutorial..ur website page is showing the description of table per class hierarchy…but the code and mapping files are proper.
It would be really nice if there was a print option so that I could print this out :-(
Hi All,
Are we using ‘discriminator’ in this example?
Pls correct me, if I am wrong.
Regards
Bala S
Where is the discriminator column you speak of.
I have a question, what if (in your example layout) i have a person already stored in db and i want that person to be an owner or an employee, it was neither before, but i want it to be now.
Im getting constraint errors when persisting or merging… how can i accomplish this scenario?
Discriminator tag is missing in the hbm file.
Otherwise excellent tutorial!
Good tutorial !!
how to map if “Employee” table has a composite primary key on it?
Hi Viral
I am a ECM Consultant and working on a applicaiton which is build on FileNet (ECM product) j2ee and hibernate.
Appreciate your help in one of the issues i am facing. I am unable to save blob to database using hibernate.
While commiting it throws error :
Exception in thread “main” java.lang.AbstractMethodError:
oracle.jdbc.driver.T2CPreparedStatement.setBinaryStream(ILjava/io/InputStream;)V
I am using ojdbc14.jar and also tried with ojdbc6.jar , not sure what is going on
Code is
session = HibernateUtil.getAuomationSessionFactory().openSession();
Transaction trans = session.beginTransaction();
ByteArrayOutputStream buffer = new ByteArrayOutputStream();
int nRead;
stream = new FileInputStream(“C:\\Upendra\\Development\\ADL CITY\\Test.txt”);
byte[] data1 = new byte[16384];
data1 = IOUtils.toByteArray(stream);
byte[] data = new byte[16384];
while ((nRead = stream.read(data, 0, data.length)) != -1) {
buffer.write(data, 0, nRead);
}
buffer.flush();
Blob blob = session.getLobHelper().createBlob(buffer.toByteArray());
emailattach.setFILENAME(“Test1.tif”);
emailattach.setFILEBLOB(blob);
session.save(emailattach);
// session.flush();
trans.commit();
Thanks very much for your attention to detail and understanding that when a person is researching something from scratch they need a simple good example. I went through a lot of pages before finding this clear concise explanation and example.
Much much thanks.
This is very useful and helped me to understand hibernate mappings and inheritance.
NEED To REMOVE::
tag is used to define the discriminator column.
tag is used to map the subclass Employee. Note that we have not used the usual tag to map Employee as it falls below in the hierarchy tree.
Nice tutorials.
nice tutorial,its really helpful .especially output database schema diagram.is easy to get the things.
Hi, Excellent tutorial. I’m new in Hibernate and it is very helpful. What happens when the owner is employee too? How to make that? Thanks
I implemented this approach, works fine as expected but I am having problems in fetching the record.
So, when I do session.get() what kind of POJO object it would get ? the Parent one or child one ?
Example:
Implementing this as Table per sub class inheritance, two tables Vehicle and Car resp.
Doing a save on vehicle object will save in Vehicle table and saving car will save in Vehicle and Car table.
But now how to fetch each respectively ??
Hi Viral,
I am using InheritanceType.Joined in one of my project.
But when i am trying to update the data using HQL query it gives error that
Sorry, In my previous comment i forgot to put the query generated by hibernate.
Below i give the sample program whih i used in my project
Class A
Class B
Class C
For updating Class B value i used below query.
I dint think that for JOINED strategy we need to put any descriminator column.
Ok – i like this approach, but i have the following question.
The information about a child can be directly removed from the database, thus causing us to have parents with no child – could this be an issue in the design?
In your example – that means having persons who is not an employee or owner.
Are you aware of something called: “Cascade.ALL” ?
Hi Viral,
Thanks for sharing the concept about Inheritance. I have a doubt. PFB my query:
Suppose If I want to add primary key in the table of child class then how I can add?
Thanks,
Sandeep
It is not at all required to add any primary key in the child table if we have in parent one, because it will mapped to the parent table PK as we mentioned in the model as putting the @PrimaryKeyJoinColumn(name=”PERSON_ID”)
You need to fix this DISCRIMINATOR
Hi,
My concern is how does private fields of super class inherit to sub class. I am not getting this plz anyone help me.
In this tutorial , you have mentioned about Discriminator column. I think, this is wrong.
The same doesn’t appear in mapping file (Person.hbm.xml) & tables too. which is quite obvious.
I think,you have wrongly pasted this from previous seeion: table per hierarchy.
Please look-into this