Welcome to Hibernate Tutorial Series. In previous tutorials we saw how to implement Inheritance in Hibernate: One Table per Subclass.
Today we will see how to implement Hibernate Inheritance: One Table per Concrete Class scheme.
Introduction to Inheritance in Hibernate
Java is an object oriented language. It is possible to implement Inheritance in Java. Inheritance is one of the most visible facets of Object-relational mismatch. Object oriented systems can model both “is a” and “has a” relationship. Relational model supports only “has a” relationship between two entities. Hibernate can help you map such Objects with relational tables. But you need to choose certain mapping strategy based on your needs.
One Table per Concrete Class example
Suppose we have a class Person with subclasses Employee and Owner. Following the class diagram and relationship of these classes.
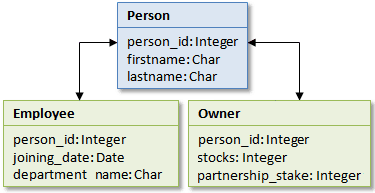
In One Table per Concrete class scheme, each concrete class is mapped as normal persistent class. Thus we have 3 tables; PERSON, EMPLOYEE and OWNER to persist the class data. In this scheme, the mapping of the subclass repeats the properties of the parent class.
Following are the advantages and disadvantages of One Table per Subclass scheme.
Advantages
- This is the easiest method of Inheritance mapping to implement.
Disadvantages
- Data thats belongs to a parent class is scattered across a number of subclass tables, which represents concrete classes.
- This hierarchy is not recommended for most cases.
- Changes to a parent class is reflected to large number of tables
- A query couched in terms of parent class is likely to cause a large number of select operations
This strategy has many drawbacks (esp. with polymorphic queries and associations) explained in the JPA spec, the Hibernate reference documentation, Hibernate in Action, and many other places. Hibernate work around most of them implementing this strategy using SQL UNION queries. It is commonly used for the top level of an inheritance hierarchy:
Create Database Table to persist Concrete classes
CREATE TABLE `person` (
`person_id` BIGINT(20) NOT NULL AUTO_INCREMENT,
`firstname` VARCHAR(50) NOT NULL DEFAULT '0',
`lastname` VARCHAR(50) NOT NULL DEFAULT '0',
PRIMARY KEY (`person_id`)
)
CREATE TABLE `employee` (
`person_id` BIGINT(10) NOT NULL AUTO_INCREMENT,
`firstname` VARCHAR(50) NOT NULL,
`lastname` VARCHAR(50) NOT NULL,
`joining_date` DATE NULL DEFAULT NULL,
`department_name` VARCHAR(50) NULL DEFAULT NULL,
PRIMARY KEY (`person_id`)
)
CREATE TABLE `owner` (
`person_id` BIGINT(20) NOT NULL AUTO_INCREMENT,
`firstname` VARCHAR(50) NOT NULL DEFAULT '0',
`lastname` VARCHAR(50) NOT NULL DEFAULT '0',
`stocks` BIGINT(11) NULL DEFAULT NULL,
`partnership_stake` BIGINT(11) NULL DEFAULT NULL,
PRIMARY KEY (`person_id`)
)
Code language: SQL (Structured Query Language) (sql)
Project Structure in Eclipse
For XML mapping
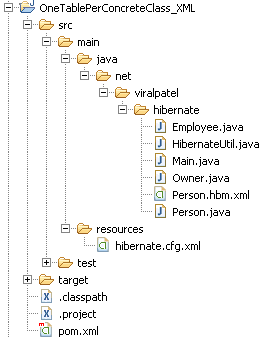
For Annotation mapping
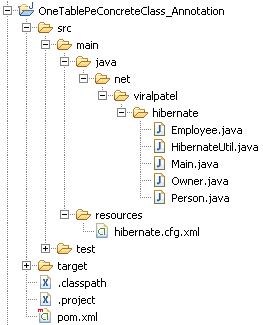
Hibernate Inheritance: XML Mapping
Following is the example where we map Person, Employee and Owner entity classes using XML mapping.
File: Person.java
package net.viralpatel.hibernate;
public class Person {
private Long personId;
private String firstname;
private String lastname;
// Constructors and Getter/Setter methods,
}
Code language: Java (java)
File: Employee.java
package net.viralpatel.hibernate;
import java.util.Date;
public class Employee extends Person {
private Date joiningDate;
private String departmentName;
// Constructors and Getter/Setter methods,
}
Code language: Java (java)
File: Owner.java
package net.viralpatel.hibernate;
public class Owner extends Person {
private Long stocks;
private Long partnershipStake;
// Constructors and Getter/Setter methods,
}
Code language: Java (java)
File: Person.hbm.xml
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE hibernate-mapping PUBLIC
"-//Hibernate/Hibernate Mapping DTD 3.0//EN"
"http://hibernate.sourceforge.net/hibernate-mapping-3.0.dtd">
<hibernate-mapping package="net.viralpatel.hibernate">
<class name="Person" table="PERSON">
<id name="personId" column="PERSON_ID">
<generator class="native" />
</id>
<property name="firstname" />
<property name="lastname" column="lastname" />
</class>
<class name="Employee">
<id name="personId" column="PERSON_ID">
<generator class="native" />
</id>
<property name="firstname" />
<property name="lastname" column="lastname" />
<property name="departmentName" column="department_name" />
<property name="joiningDate" type="date" column="joining_date" />
</class>
<class name="Owner">
<id name="personId" column="PERSON_ID">
<generator class="native" />
</id>
<property name="firstname" />
<property name="lastname" column="lastname" />
<property name="stocks" column="stocks" />
<property name="partnershipStake" column="partnership_stake" />
</class>
</hibernate-mapping>
Code language: HTML, XML (xml)
Note that we have defined only one hibernate mapping (hbm) file Person.hbm.xml
. Both Person
and Employee
model class are defined within one hbm file.
We mapped all the classes using simple <class> tag in hbm. This is the usual way of mapping classes in XML.
Hibernate Inheritance: Annotation Mapping
Following is the example where we map Person, Employee and Owner entity classes using JPA Annotations.
File: Person.java
package net.viralpatel.hibernate;
import javax.persistence.Column;
import javax.persistence.Entity;
import javax.persistence.Id;
import javax.persistence.Inheritance;
import javax.persistence.InheritanceType;
import javax.persistence.Table;
@Entity
@Table(name = "PERSON")
@Inheritance(strategy = InheritanceType.TABLE_PER_CLASS)
public class Person {
@Id
@Column(name = "PERSON_ID")
private Long personId;
@Column(name = "FIRSTNAME")
private String firstname;
@Column(name = "LASTNAME")
private String lastname;
public Person() {
}
public Person(String firstname, String lastname) {
this.firstname = firstname;
this.lastname = lastname;
}
// Getter and Setter methods,
}
Code language: Java (java)
@Inheritance
– Defines the inheritance strategy to be used for an entity class hierarchy. It is specified on the entity class that is the root of the entity class hierarchy.
@InheritanceType
– Defines inheritance strategy options. TABLE_PER_CLASS is a strategy to map table per concrete class.
File: Employee.java
package net.viralpatel.hibernate;
import java.util.Date;
import javax.persistence.AttributeOverride;
import javax.persistence.AttributeOverrides;
import javax.persistence.Column;
import javax.persistence.Entity;
import javax.persistence.Table;
@Entity
@Table(name="EMPLOYEE")
@AttributeOverrides({
@AttributeOverride(name="firstname", column=@Column(name="FIRSTNAME")),
@AttributeOverride(name="lastname", column=@Column(name="LASTNAME"))
})
public class Employee extends Person {
@Column(name="joining_date")
private Date joiningDate;
@Column(name="department_name")
private String departmentName;
public Employee() {
}
public Employee(String firstname, String lastname, String departmentName, Date joiningDate) {
super(firstname, lastname);
this.departmentName = departmentName;
this.joiningDate = joiningDate;
}
// Getter and Setter methods,
}
Code language: Java (java)
File: Owner.java
package net.viralpatel.hibernate;
import javax.persistence.AttributeOverride;
import javax.persistence.AttributeOverrides;
import javax.persistence.Column;
import javax.persistence.Entity;
import javax.persistence.Table;
@Entity
@Table(name="OWNER")
@AttributeOverrides({
@AttributeOverride(name="firstname", column=@Column(name="FIRSTNAME")),
@AttributeOverride(name="lastname", column=@Column(name="LASTNAME"))
})
public class Owner extends Person {
@Column(name="stocks")
private Long stocks;
@Column(name="partnership_stake")
private Long partnershipStake;
public Owner() {
}
public Owner(String firstname, String lastname, Long stocks, Long partnershipStake) {
super(firstname, lastname);
this.stocks = stocks;
this.partnershipStake = partnershipStake;
}
// Getter and Setter methods,
}
Code language: Java (java)
Both Employee and Owner classes are child of Person class. Thus while specifying the mappings, we used @AttributeOverrides
to map them.
@AttributeOverrides
– This annotation is used to override mappings of multiple properties or fields.
@AttributeOverride
– The AttributeOverride annotation is used to override the mapping of a Basic (whether explicit or default) property or field or Id property or field.
The AttributeOverride annotation may be applied to an entity that extends a mapped superclass or to an embedded field or property to override a basic mapping defined by the mapped superclass or embeddable class. If the AttributeOverride annotation is not specified, the column is mapped the same as in the original mapping.
Thus in over case, firstname and lastname are defined in parent class Person and mapped in child class Employee and Owner using @AttributeOverrides annotation.
This strategy supports one-to-many associations provided that they are bidirectional. This strategy does not support the IDENTITY generator strategy: the id has to be shared across several tables. Consequently, when using this strategy, you should not use AUTO nor IDENTITY. Note that in below Main class we specified the primary key explicitly.
Main class
package net.viralpatel.hibernate;
import java.util.Date;
import org.hibernate.Session;
import org.hibernate.SessionFactory;
public class Main {
public static void main(String[] args) {
SessionFactory sf = HibernateUtil.getSessionFactory();
Session session = sf.openSession();
session.beginTransaction();
Person person = new Person("Steve", "Balmer");
person.setPersonId(1L);
session.save(person);
Employee employee = new Employee("James", "Gosling", "Marketing", new Date());
employee.setPersonId(2L);
session.save(employee);
Owner owner = new Owner("Bill", "Gates", 300L, 20L);
owner.setPersonId(3L);
session.save(owner);
session.getTransaction().commit();
session.close();
}
}
Code language: Java (java)
The Main class is used to persist Person
, Employee
and Owner
object instances. Note that these classes are persisted in different tables and parent attributes (firstname, lastname) are repeated across all tables.
Output
Hibernate: insert into PERSON (FIRSTNAME, LASTNAME, PERSON_ID) values (?, ?, ?)
Hibernate: insert into EMPLOYEE (FIRSTNAME, LASTNAME, department_name, joining_date, PERSON_ID) values (?, ?, ?, ?, ?)
Hibernate: insert into OWNER (FIRSTNAME, LASTNAME, partnership_stake, stocks, PERSON_ID) values (?, ?, ?, ?, ?)
Code language: SQL (Structured Query Language) (sql)
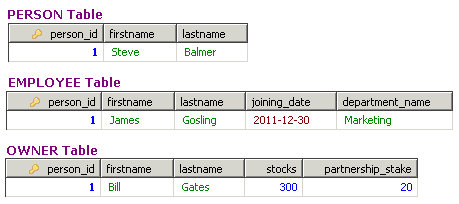
Download Source Code
Hibernate-inheritance-table-per-concrete-class_xml.zip (9 KB)
Hibernate-inheritance-table-per-concrete-class_annotation.zip (9 KB)
hi viral,
good article.
Thanks :)
Good Job and great explained Mr. Viral.
Thanks A Lot
:-)
vipul sir,
the HIBERNATE phrases say meaning clearly 1> table per subclass(only subclasses store infomation no super class table)
2>table per Concrete class——(only object existed classes posses database table)
but in ur 2 examples both scenarios has 3 tables(base- derived-derived) its a bit confusing
can u put just theritical explanation on this.
hi sir u have done grate job.
Thanks Viral, for good explanation
Thanks Viral its great really its very very useful
awesome tutorial .!! thanks .!!
Good Article……. for hibernate inheritance
nice explanation thanQ u viral
I don’t see repetition of data.. for example… James and Bill are not appearing in the Person table
Its very nice & almost very useful for everyone especially for beginners.
awesome :P
Thanks for u r explanation .it helps me alot to resolve my issues.
U r setting the code as followings:
person.setPersonId(1L);
employee.setPersonId(2L);
owner.setPersonId(3L);
For which the output is different in which everywhere its showing the id as 1 in all the three tables.
I second Varun, i noticed the same issue. Something is wrong.
There is problem with primary key. Its not constant in all the three tables as you have shown in the tutorial.
I think the result db image is wrong, there whould be 3 rows in Person don’t you think? because you inserted 1 of each kind of data (three), 1 Person, 1 employee (Person), 1 Owner (Person)… and 1 row in the other tables…
The correct way would be NOT to override the fields.
I dont see how data is scattered in other tables, the design is normalized when holding data specific to each table/class. The employee has a name, becuase the employee is a person that has a name.
It was a great tutorial. Thank you very much!!! :)
My mistake, i confused JOINED withe SINGLE_TABLE…. XD
nice tut
short and to the point Viral.
I am trying to import this project into my workspace but it complains about –
An internal error occurred during: “Importing Maven projects”.
Unsupported IClasspathEntry kind=4
The reason to import being – i wanted a working inheritance example using mysql.
I am tryin to have something like this –
and child class which for now doesn’t have any attributes of its own but only parent’s
I’m afraid it gives me some kind of id generation error even though i have GenerationType.IDENTITY
Any advice??
works for Display User/Employees. when i change @GeneratedValue (strategy=GenerationType.IDENTITY) in User class to @GeneratedValue (strategy=GenerationType.TABLE).
But, now when I add a User it gives me ‘hibernate_sequences’ error.
———————————————–
————————————————–
Facing the error:
Exception in thread “Main Thread” org.hibernate.AnnotationException: No identifier specified for entity: com.st.vo.SalariedEmployee
at org.hibernate.cfg.InheritanceState.determineDefaultAccessType(InheritanceState.java:268)
at org.hibernate.cfg.InheritanceState.getElementsToProcess(InheritanceState.java:223)
at org.hibernate.cfg.AnnotationBinder.bindClass(AnnotationBinder.java:686)
at org.hibernate.cfg.Configuration$MetadataSourceQueue.processAnnotatedClassesQueue(Configuration.java:3977)
at org.hibernate.cfg.Configuration$MetadataSourceQueue.processMetadata(Configuration.java:3931)
at org.hibernate.cfg.Configuration.secondPassCompile(Configuration.java:1368)
at org.hibernate.cfg.Configuration.buildSessionFactory(Configuration.java:1826)
at com.st.utils.HibernateUtils.getSessionFactory(HibernateUtils.java:14)
at com.st.vo.TablePerClassTestCase.main(TablePerClassTestCase.java:14)
Facing the above error even after providing @identifier …….
@Santosh: Can you add
generate=GeneratorType.TABLE
to @Id definition inEmployee
class?@Id(generate=GeneratorType.TABLE)
Hi Viral,
Nice article, But i found many copy paste issue in the these article. Though they are easy to avoid but for beginers, it is little confusing.
Thanks for sharing.
nice examle:
i have same senario example
in my domain classess i have, person class as abstract class, so no table will be created for person class, i have a case that a person that can be owner and employee at same time, and class owner has set of employees work for that owner.
owner.class
Hey,
Thanks for a good article.
I just have one concern that when using TABLE_PER_CLASS strategy, do we need to have the physical “Person” table in the database?
Good and well explained tutorial for Hibernate
Many tutorial are available but this tutorial for Hibernate mapping and Hibernate inheritence concept point of view, I would say it is nice and no comparision at all.
@Viral Patel,
Please provide us “CURD based Hibernate-Spring” tutorial via using HibernateDAO and HibernateTemplate and HibernateFactory using SessionFactory to do all CURD related functionality to and from MySQL/Oracle database.
@Viral Patel,
Sir Why you are not providing Spring tutorial like this as Hibernate have (very selected but nicely explained and good one) as there are so many tutorial but Viral Patel sir something we are missing which you can fulfill by providing “Spring Tutorial” for many people in india and abroad.
Only MkYong is there but you can provide something more than that when you will come into Spring also so please provide some selected great topic relates to Spring Core and Spring MVC also.
Check the Spring MVC series of tutorials: http://viralpatel.net/tutorial-spring-3-mvc-introduction-spring-mvc-framework/ Might be useful :)
Hi Viral
I am getting an error for the “Hibernate Inheritance: Table Per Concrete Class (Annotation & XML mapping)” example… The error is as below…
Note: I am using Hibernate 3.6 and mysql 5.5
Exception in thread “main” org.hibernate.exception.GenericJDBCException: could not insert: [com.viralpatel.tableperconcreteclass.Employee]
Caused by: java.sql.SQLException: Field ‘DISCRIMINATOR’ doesn’t have a default value
Please assist, if possible..
Regards
Nadeem
Fantastic article, Viral. Very well written, clear and concise. One of the best links explaining one to many and hierarchy in hibernate. Thanks a lot.
it is explained in a nice way. Keep it up, Viral.
Hi Viral,
Thanks for giving Good Example.
I have one problem with the update.
EX: If i try to update Owner using update query it fails because of inheritance issue.
Can you please provide some suggetions?
thanks
Very neat and clear examples.Thanks
Can we declare person class as abstract in case if our application is not going to have any person instance rather will have only a sub class instance as employee or owner etc?
Very good Tutorial very clean explanation thank you sir .This post is very help full for me
hey,too cool stuff..
very much thanks to you :-)
i got this exception :- An entity annotated with @Inheritance cannot use @AttributeOverride or @AttributeOverrides: net.viralpatel.hibernate.Employee
what i do…?