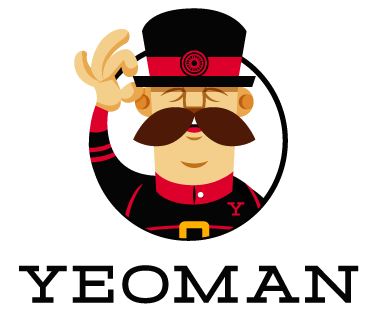
Yeoman is a Node module to automate your front-end project build process, so that all we as a developer only need to worry about is to code and test. Think of it as a Maven for your next JS based project. It does scaffolding of your project, manage your dependencies and then does the building. To perform these tasks, Yeoman is made up of three tools:
- yo: For scaffolding the application. That means you don’t worry about creating all those directory structures, writing basic JS file to get a project up and running. It magically creates all directory as per industry best practice, along with executable code to get the application up.
- bower: Managing all JS/CSS based dependencies. So next time when you need to include UI-Router in your angular JS application, instead of manually downloading the latest JS file, just update the bower config file and run
bower install
command. - grunt: Building the project to make it production ready. This may include running JSHint, Test Cases, Minifying your JS, CSS files, Uglifying JS files and many other plugin based features.
Let’s Get Started
Since Yeoman comes as a Node module, before we can install Yeoman make sure you have Node installed on your system. If not, it’s a good time to install Node as most of industries front-end framework are now available as a Node Module, and it’s really easy to install new modules once you have Node. To install Node, just go to http://nodejs.org/ and follow the Install instruction.
Back to Business
Assuming you now have Node and Node Package Manager (NPM) installed (NPM automatically gets installed along with Node), let’s install Yeoman. To do so use below command:
npm install -g yo
or if you don’t have sufficient permission, you may need to run it using sudo
:
sudo npm install -g yo
SideNote: If you are behind a proxy, you might need to first configure npm
npm set config proxy http://proxy-address.com:8000
npm set config https-proxy https://proxy-address.com:8000
Code language: JavaScript (javascript)
After installation, before you can scaffold the application we need to install Yeoman Generators. Yeoman scaffolds different types of application through different Generator (which again are distributed through npm). So for example, if you want to start a AngularJs application you will install generator-angular using npm. There are many Official and Community based generators available, or if you don’t find one which you need… you can create a Generator of your own.
Let’s create a simple AngularJs and Twitter Bootstrap based application
First, install Angular-Bootstrap Generator:
npm install -g generator-angular-bootstrap
You will see many GET and REDIRECT requests. On completion, you will see a message like below:
generator-angular-bootstrap@0.4.3 /usr/local/lib/node_modules/generator-angular-bootstrap
├── semver@2.3.1
├── bluebird@2.2.2
├── needle@0.7.2 (qs@0.6.6, iconv-lite@0.4.3)
└── yeoman-generator@0.17.1 (dargs@0.1.0, github-username@0.1.1, diff@1.0.8, class-extend@0.1.1, rimraf@2.2.8, text-table@0.2.0, chalk@0.4.0, mime@1.2.11, async@0.9.0, isbinaryfile@2.0.1, debug@1.0.3, nopt@3.0.1, grouped-queue@0.3.0, shelljs@0.3.0, mkdirp@0.5.0, glob@4.0.4, findup-sync@0.1.3, underscore.string@2.3.3, iconv-lite@0.2.11, download@0.1.18, file-utils@0.2.0, request@2.37.0, lodash@2.4.1, gruntfile-editor@0.1.1, cheerio@0.17.0, inquirer@0.5.1)
Code language: JavaScript (javascript)
All Set! Create Yeoman Project
Ok great, we have Yeoman! and we have its AngularJs-Bootstrap Generator! Let’s kick-start our project.
Create a project directory, name it ‘YoProject’.
mkdir YoProject cd YoProject
In this new directory, just execute below “yo” command:
yo angular-bootstrap
During executing of above command, Yeoman will ask you couple of questions required to set up your project. I answered them as below:
[?] What version of angular would you like to use? 1.3.0-beta.15
[?] Which official angular modules would you need? animate, resource, route
[?] Any third-party component you might require? twbs/bootstrap (3.2.0), fortawesome/font-awesome (4.1.0), angular-ui/ui-router (0.2.10)
[?] What build system would I use? grunt
[?] Should I set up one of those HTML preprocessors for you? none
[?] Should I set up one of those JS preprocessors for you? none
[?] Should I set up one of those CSS preprocessors for you? sass
[?] Would you want me to support old versions of Internet Explorer (eg. before IE9)? no
[?] What's the base name of your project? YoProject
Code language: JavaScript (javascript)
After answering all question, it will download required libraries and scaffold the application. Once done check the directory content, you will see that every thing you need to get started is already there.
aadi@ubuntu:~/eclipse_ws/YoProject$ tree .
.
+-- app
¦ +-- index.html
¦ +-- scripts
¦ ¦ +-- app.js
¦ ¦ +-- controllers
¦ ¦ ¦ +-- main.js
¦ ¦ +-- directives
¦ ¦ ¦ +-- sample.js
¦ ¦ +-- filters
¦ ¦ ¦ +-- sample.js
¦ ¦ +-- services
¦ ¦ +-- sample.js
¦ +-- styles
¦ ¦ +-- main.css
¦ +-- views
¦ +-- contact.html
¦ +-- features.html
¦ +-- home.html
¦ +-- partials
¦ +-- footer.html
¦ +-- header.html
+-- bower.json
+-- Gruntfile.js
+-- npm-debug.log
+-- package.json
+-- README.md
9 directories, 17 files
Code language: JavaScript (javascript)
Once your application is created, install bower dependencies using:
bower install
These dependencies are downloaded under app/bower_components directory. Next, you can run below grunt command to include these dependencies in index.html file.
aadi@ubuntu:~/eclipse_ws/YoProject$ grunt bowerInstall
Running "bowerInstall:app" (bowerInstall) task
app/index.html modified.
Done, without errors.
Code language: JavaScript (javascript)
This command updates the index.html file to include all the dependencies. After change, my index.html
file has these new lines:
....
<!-- build:css styles/components.min.css -->
<!-- bower:css -->
<link rel="stylesheet" href="bower_components/bootstrap/dist/css/bootstrap.css" />
<link rel="stylesheet" href="bower_components/font-awesome/css/font-awesome.css" />
<!-- endbower -->
<!-- endbuild -->
....
<!-- build:js scripts/components.min.js -->
<!-- bower:js -->
<script src="bower_components/jquery/dist/jquery.js"></script>
<script src="bower_components/angular/angular.js"></script>
<script src="bower_components/angular-ui-router/release/angular-ui-router.js"></script>
<script src="bower_components/angular-animate/angular-animate.js"></script>
<script src="bower_components/angular-resource/angular-resource.js"></script>
<script src="bower_components/angular-route/angular-route.js"></script>
<!-- endbower -->
....
Code language: HTML, XML (xml)
You can now open up ‘app/index.html’ in browser and see application is up and running and you are all set to start making changes.
You may take a look at the code generated using above steps on GitHub.
To cut-short above process, we first installed Yeoman and its angular-bootstrap Generator. Then we scaffolded the application using ‘yo angular-bootstrap’, installed its dependencies using ‘bower install’ and then built is using ‘grunt bowerInstall’. Once familiar, this process becomes very easy to kickstart and maintain projects as compared to downloading individual dependencies and updating index.html each time.
There are many interesting and powerful generator available which can build and manage not only client side of application but also full application stack. For example, an application with Stack as ‘Twitter Bootstap – AngularJs – NodeJs – ExpressJS – MongooseJs – MongoDB’ can be generated using a popular community generator: generator-angular-fullstack
That’s it, hope that was helpful!
nice. thanks
Nice Tutorial………….
good introductory tutorial
I am getting “template render” error unknown path
I fixed it by down grading the [email protected] following this stackoverflow link http://stackoverflow.com/questions/26580403/error-when-using-yeoman-to-generate-angular-app-unhandled-template-render-error.
Thank for the awesome tutorial as usual.
Very nice introduction to yeoman tool