Nowadays, Quick Response (QR) Codes are becoming more and more useful as they have gone mainstream, thanks to the smart phones. Right from the bus shelter, product packaging, home improvement store, automobile, a lot of internet websites are integrating QR Codes on their pages to let people quickly reach them. With increase in number of users of smart phones day by day, the QR codes usage is going up exponentially.
Let us see a quick overview of Quick Response (QR) codes and also how to generate these codes in Java.
Introduction to QR Codes
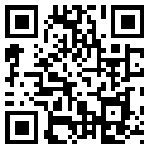
A QR code (abbreviated from Quick Response code) is a type of matrix barcode (or two-dimensional code) first designed for the automotive industry. More recently, the system has become popular outside of the industry due to its fast readability and comparatively large storage capacity. The code consists of black modules arranged in a square pattern on a white background. The information encoded can be made up of four standardised kinds (“modes”) of data (numeric, alphanumeric, byte/binary, Kanji), or by supported extensions virtually any kind of data.
Created by Toyota subsidiary Denso Wave in 1994 to track vehicles during the manufacturing process, the QR code is one of the most popular types of two-dimensional barcodes. It was designed to allow its contents to be decoded at high speed.
Hello World QR Code in Java
Zebra Crossing (ZXing) is an awesome open source library that one can use to generate / parse QR Codes in almost all the platforms (Android, JavaSE, IPhone, RIM, Symbian etc). But if you have to generate simple QR Codes, I found it a bit clumsy to implement.
However QRGen is a good library that creates a layer on top of ZXing and makes QR Code generation in Java a piece of cake. It has a dependency on ZXing, so you would need ZXing jar files along with QRGen to create QR Codes in Java.
On the download page of ZXing, you will not find the JAR files. Instead we have to create JAR files using the source code. I have already generated these JAR files.
Here are the links:
zxing-core-1.7.jar (346 KB)
zxing-javase-1.7.jar (21 KB)
Also download the QRGen JAR File from their download page.
Include these JAR files in your Classpath and execute following Java code to generate QR Code.
package net.viralpatel.qrcode;
import java.io.ByteArrayOutputStream;
import java.io.File;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.IOException;
import net.glxn.qrgen.QRCode;
import net.glxn.qrgen.image.ImageType;
public class Main {
public static void main(String[] args) {
ByteArrayOutputStream out = QRCode.from("Hello World")
.to(ImageType.PNG).stream();
try {
FileOutputStream fout = new FileOutputStream(new File(
"C:\\QR_Code.JPG"));
fout.write(out.toByteArray());
fout.flush();
fout.close();
} catch (FileNotFoundException e) {
// Do Logging
} catch (IOException e) {
// Do Logging
}
}
}
Code language: Java (java)
The code is pretty straight forward. We used QRCode
class to generate QR Code Stream and write the byte stream to a file C:\QR_Code.JPG.
Download Source Code
QR_Code_Java.zip (339 KB)
If you open this JPEG file and scan using your iPhone or Android QR scanner, you’ll find a cool “Hello World” in it :)
Apart from generating Sterams of data using QRGen API, we can also use below APIs to create QR Codes:
// get QR file from text using defaults
File file = QRCode.from("Hello World").file();
// get QR stream from text using defaults
ByteArrayOutputStream stream = QRCode.from("Hello World").stream();
// override the image type to be JPG
QRCode.from("Hello World").to(ImageType.JPG).file();
QRCode.from("Hello World").to(ImageType.JPG).stream();
// override image size to be 250x250
QRCode.from("Hello World").withSize(250, 250).file();
QRCode.from("Hello World").withSize(250, 250).stream();
// override size and image type
QRCode.from("Hello World").to(ImageType.GIF).withSize(250, 250).file();
QRCode.from("Hello World").to(ImageType.GIF).withSize(250, 250).stream();
Code language: Java (java)
Website Link (URLs) QR Code in Java
One of the most common use of a QR Code is to bring traffic to a particular webpage or download page of website. Thus QR Code encodes a URL or website address which a user can scan using phone camera and open in their browser. URLs can be straight forward included in QR Codes. In above Java Hello World example, just replace “Hello World” string with the URL you want to encode in QR Code. Below is the code snippet:
ByteArrayOutputStream out = QRCode.from("//www.viralpatel.net")
.to(ImageType.PNG).stream();
Code language: Java (java)
QR Code in Servlet
Most of the time you would need to generate QR Codes dynamically in some website. We already saw how easy it is to generate QR code in Java. Now we will see how to integrate this QR Code generation in a Java Servlet.
Following is a simple Http Servlet that creates QR Code using QRGen and ZXing library. User provides the text for which QR Code is generated.
The index jsp file contains a simple html form with a textbox and submit button. User can enter the text that she wishes to generate QR code of and presses submit.
File: index.jsp
<html>
<head>
<title>QR Code in Java Servlet - viralpatel.net</title>
</head>
<body>
<form action="qrservlet" method="get">
<p>Enter Text to create QR Code</p>
<input type="text" name="qrtext" />
<input type="submit" value="Generate QR Code" />
</form>
</body>
</html>
Code language: HTML, XML (xml)
The magic happens in QRCodeServlet.java
. Here we uses QRGen library along with ZXing and generates QR Code for given text (Text we get from request.getParameter). Once the QR Stream is generated, we write this to response and set appropriate content type.
File: QRCodeServlet.java
package net.viralpatel.qrcodes;
import java.io.ByteArrayOutputStream;
import java.io.File;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.OutputStream;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import net.glxn.qrgen.QRCode;
import net.glxn.qrgen.image.ImageType;
public class QRCodeServlet extends HttpServlet {
@Override
protected void doGet(HttpServletRequest request,
HttpServletResponse response) throws ServletException, IOException {
String qrtext = request.getParameter("qrtext");
ByteArrayOutputStream out = QRCode.from(qrtext).to(
ImageType.PNG).stream();
response.setContentType("image/png");
response.setContentLength(out.size());
OutputStream outStream = response.getOutputStream();
outStream.write(out.toByteArray());
outStream.flush();
outStream.close();
}
}
Code language: Java (java)
The below web.xml simply maps QRCodeServlet.java
with /qrservlet
URL.
File: web.xml
<?xml version="1.0" encoding="UTF-8"?>
<web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns="http://java.sun.com/xml/ns/javaee"
xmlns:web="http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd"
xsi:schemaLocation="http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd"
id="WebApp_ID" version="2.5">
<display-name>QR_Code_Servlet</display-name>
<welcome-file-list>
<welcome-file>index.jsp</welcome-file>
</welcome-file-list>
<servlet>
<servlet-name>QRCodeServlet</servlet-name>
<servlet-class>net.viralpatel.qrcodes.QRCodeServlet</servlet-class>
</servlet>
<servlet-mapping>
<servlet-name>QRCodeServlet</servlet-name>
<url-pattern>/qrservlet</url-pattern>
</servlet-mapping>
</web-app>
Code language: HTML, XML (xml)
Download Source Code
QR_Code_Servlet.zip (340 KB)
Output
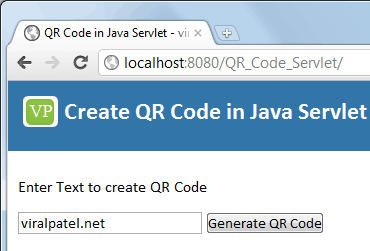
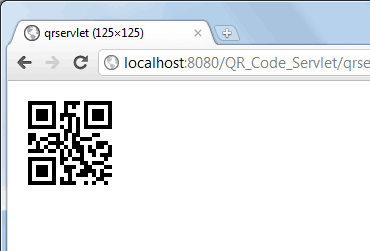
Conclusion
Generating QR Codes in Java is not only easy, but quite straight forward. Integrating this functionality with any existing Java based app is just a piece of cake! In this tutorial we saw how to generate these QR codes in Java and also with Servlet.
Hope you’ll like this :)
Good QR code tutorial
Java Programming
The hyperlink for the servlet source code is incorrect. It points to the same zip file as for the Java code example. The correct URL should be http://viralpatel-net-tutorials.googlecode.com/files/QR_Code_Servlet.zip
Gerry, this is the second time you catching up the typos on my articles :) Thanks a ton mate!!! I’ve updated the link.
Hi, how to start this project.
The netbeans or eclipse?
Here is a wrapper for using Google Charts QR-codes: http://www.servletsuite.com/servlets/qrcodepackage.htm
nice one!
Good one
im using the 3 .JAR files in my blackberry project..
ive copied them into a new “lib” folder in my project folder..
then ive added them to the build path in thru project properties..
the classes are recognized whil coding but i get this when packaging:
————————————————————————————————————————-
“Packaging project QR_Generator
C:\Eclipse\plugins\net.rim.ejde.componentpack5.0.0_5.0.0.25\components\bin\rapc.exe -quiet codename=deliverables\Standard\5.0.0\QR_Generator deliverables\Standard\5.0.0\QR_Generator.rapc -sourceroot=C:\Blackberry\workspace\QR_Generator\src;C:\Blackberry\workspace\QR_Generator\res -import=C:\Eclipse\plugins\net.rim.ejde.componentpack5.0.0_5.0.0.25\components\lib\net_rim_api.jar;C:\Blackberry\workspace\QR_Generator\lib\qrgen-1.0.jar;C:\Blackberry\workspace\QR_Generator\lib\zxing-core-1.7.jar;C:\Blackberry\workspace\QR_Generator\lib\zxing-j2se-1.7.jar C:\Blackberry\workspace\QR_Generator\bin
net/glxn/qrgen/exception/QRGenerationException.class: Error!: Invalid class file: Incorrect classfile version
Packaging project QR_Generator failed (took 1.12 seconds) ”
————————————————————————————————————————-
useful stuff…..
Thanks
Now what….lets go for the next step…..where I can found some info about QR “READER”….I would like to develop a program for smartphones, specifically for my nokia symbian phone, Id like to have some help in this because I have some personal use for this qr reader custom-made!!Thanks for the help!!
Thanks Viral for sharing code on QR code encoding.
Do you have similar code for QR code decoding?
Can you please share it with me
I’ve only java 1.4.2 in my project..pls suggest
This is good and useful.
Can anyone tell me how to a add muitlple data in the QR code .
Like Name,Phone number,website url ………..
Appreciate some sample code :-)
it didn’t work with me no errors it run but this out put don’t appear plz help me quickly
java.lang.NoClassDefFoundError: java.awt.image.BufferedImage at com.google.zxing.client.j2se.MatrixToImageWriter.toBufferedImage(MatrixToImageWriter.java:48) at com.google.zxing.client.j2se.MatrixToImageWriter.writeToStream(MatrixToImageWriter.java:75)
This is the error get when i am intigrate the project with android platform
Can u please tell me about how to integrate the qr code generator file in android project. because AWT is not supported on Android OS.so please give me some tips to include qr code generator in android project
Thanks®ards
””””””””””””””””””””””’
RAMBABU
I already try the tutorial above and it works well. But, my question is what about J2ME, how to
make qrcode using zxing in j2me
thank you
Fauzi, I am looking to do the same thing – have you found a solution?
thank you
Is there a way to showtext at the bottom of the QRCode Image generated. Something like ShowText() method?
Good one…
Thanks…:)
Nice Explanation….
Is there any chance of setting the QR code Error Correction Level…
If not what is the Error Correction Level does the QR code is assigned by zxing
i’m sorry, i confused with QRCode.from because i can’t see QRCode.from in core library, is there any way to do this?
i have include all the specified libraries in my class path but when i am trying to execute the code its giving following errors:
package net.glxn.qrgen does not exist
package net.glxn.qrgen.image does not exist
please help me out…..Thanks.
Its not working for android .I am getting this error “java.lang.NoClassDefFoundError: net.glxn.qrgen.QRCode ” .Any one know solution kindly shre it with me as well
i want an example code to read qr code..thanks in advance
great Tuto
Thanks dude. it worked for me.
cant qr codes be created using C language?
excellent post! :D
can you help me , and explain how to add the jar’s file to the class path?
I have a maven project and i now i nee to add dependence to the pom.xml,
but i don’t know what to write.
Thanks’
Thnx..patel
I search all over the place to integrate this code with android.
so far the only problem is with the class awt (java.awt.* ) Its not available for android.
Is there any method by with I can integrate the code over to android,
Thanks for the tutorial :
I have downloaded the source code and unable to run those code , it says :
“qrservlet could find”
Please help
Great Post Viral.
Is there also a way to set the error correction level using this library?
thnx sir…its working…
Hi
Great Script.
I only have one problem. When I create the jar file the script doesn’t work anymore. If I run it with netbeans it works correctly but when I try to use the jar file it doesn’t work anymore
LG Laurenz
Hey, thanks for posting this. I tried your code. The compiler throw errors:
cannot access net.glxn.qrgen.QRCode
bad class file: D:\workSpace\tech\referenceClass\net\glxn\qrgen\QRCode.class
class file has wrong version 50.0, should be 48.0
Please remove or make sure it appears in the correct subdirectory of the classpath.
import net.glxn.qrgen.QRCode;
I download both qrgen-1.0.jar & qrgen-1.jar for testing. I got the above result. Am I doing something wrong?
Never mind. I find the solution to my own problem. If I use jdk1.6, it will pass the compiler. This is what I learn and would like to share with everyone:
jdk1.4 compiler error: class file has wrong version 50.0, should be 48.0
jdk1.5 compiler erro: class file has wrong version 50.0, should be 49.0
jdk1.6 compiler NO ERROR
thanks sir…can u sugest for decoding?
hi, tje code works excelent, but, how can i get a bigger image??
help, how can I open this project .
The Netbeans or Ecliese its ok
Cool article man, Helped me Just in Time :-)
Thanx
is there any way to change the color of the generated QR image?
I assume you have the problem that the QR code is red-ish when creating JPG files.
This is a bug in the MatrixToImageWriter in version 1.7 of zxing javase.
You can simply upgrade to the latest zxing version (http://code.google.com/p/zxing/) to fix the problem.
Muchas gracias, me ha servido bastante.
very nice
thanks
great!!! thanks you very much
Excellent Thx
QRCodeServlet.java:15: error: package net.glxn.qrgen does not exist
import net.glxn.qrgen.QRCode;
^
QRCodeServlet.java:16: error: package net.glxn.qrgen.image does not exist
import net.glxn.qrgen.image.ImageType;
^
QRCodeServlet.java:26: error: cannot find symbol
ImageType.PNG).stream();
^
symbol: variable ImageType
location: class QRCodeServlet
QRCodeServlet.java:25: error: cannot find symbol
ByteArrayOutputStream out = QRCode.from(qrtext).to(
^
symbol: variable QRCode
location: class QRCodeServlet
4 errors
It shows me these errors while compiling this code.
Please solve it…
really works!!!!! so helpful!!
hi,
fist thanks for this code ……. its work really good and also generate OR code.
but i want to save this QR code to the particular folder.so,plz help me and give me code for saving this image to folder.
Man, thank you so much! That’s amazing this work. I was needing to do some work and I didn’t know how I Would make it. Thanks and nice Blog.
Link to QRGen JAR is broken (1.0 version); Can be found here:
https://github.com/alyssialui/NCMS
Wow man it helps me a lot
This worked for me – thanks for the clear instructions and demonstration !
When I use and do whatever you did My browser doesnot display the qr code it instead throws an exception i.e it shows
” javax.servlet.ServletException: Servlet execution threw an exception
org.apache.tomcat.websocket.server.WsFilter.doFilter(WsFilter.java:52)
root cause
java.lang.NoClassDefFoundError: net/glxn/qrgen/QRCode
QR.QRCodeServlet.doGet(QRCodeServlet.java:18)
javax.servlet.http.HttpServlet.service(HttpServlet.java:618)
javax.servlet.http.HttpServlet.service(HttpServlet.java:725)
org.apache.tomcat.websocket.server.WsFilter.doFilter(WsFilter.java:52)
root cause
java.lang.ClassNotFoundException: net.glxn.qrgen.QRCode
org.apache.catalina.loader.WebappClassLoader.loadClass(WebappClassLoader.java:1284)
org.apache.catalina.loader.WebappClassLoader.loadClass(WebappClassLoader.java:1132)
QR.QRCodeServlet.doGet(QRCodeServlet.java:18)
javax.servlet.http.HttpServlet.service(HttpServlet.java:618)
javax.servlet.http.HttpServlet.service(HttpServlet.java:725)
org.apache.tomcat.websocket.server.WsFilter.doFilter(WsFilter.java:52)
”
What could be the problem?
integrate qr code scanner for android in c#
http://www.keepdynamic.com/dotnet-barcode-reader/qr-code.shtml
Hello!
please, i use this source code to generate QR code as servlet and it works, but i need to recuperate the image in image tag as
.
and thanks for help
Very nice examples.
Hi,
Please anyone let me know how to read a contents of a file and generate the QR codes
Thanks
Hello,i find a sample code of how to generate qr qode in java class,but i am not professional in this files,can anyone tell me is this code available?
Sample code:(http://www.keepautomation.com/products/java_barcode/barcodes/qrcode.html)
public static void main(String[] args)
{
BarCode barcode = new BarCode();
barcode.setCodeToEncode(“123456789”);
barcode.setSymbology(IBarCode.QRCODE);
barcode.setQrCodeDataMode(IBarCode.QR_MODE_AUTO);
barcode.setQrCodeEcl(IBarCode.QR_ECL_L);
barcode.setQrCodeVersion(1);
try
{ barcode.draw(“c://qrcode.gif”);
}
catch (Exception e)
{
e.printStackTrace();
}
}
it shows
” javax.servlet.ServletException: Servlet execution threw an exception”
Please help .
For those who might encounter “NoSuchMethodError”, it means the qrgen jar is not compatible with the zxing 1.7 jars.
To fix this, it’s either you
(1) get the updated zxing jars to work with the latest qrgen jar or
(2) get a lower version of qrgen to work with the zxing 1.7 jars.
I took option 2 and I can confirm qrgen-1.2.jar works with the zxing version on this page.
The QRGen download link also no longer exists, the new download page can be found at
http://search.maven.org/#browse|-852965118
The above code which you have provided is perfectly working fine with one input textbox.
What i need is to generate the QR code with more Textboxes.
Can any one help me in this regard?
Thanks in advance.
Hello Sir I want to make a card format with this qr code… Please help me
[ code java] package net.viralpatel.qrcodes;
import java.io.ByteArrayOutputStream;
import java.io.File;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.OutputStream;
import java.io.PrintWriter;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import net.glxn.qrgen.QRCode;
import net.glxn.qrgen.image.ImageType;
public class QRCodeServlet2 extends HttpServlet {
@Override
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
String qrtext = request.getParameter(“qrtext”);
ByteArrayOutputStream out = QRCode.from(qrtext).to(
ImageType.PNG).stream();
response.setContentType(“image/png”);
response.setContentLength(out.size());
OutputStream outStream = response.getOutputStream();
outStream.write(out.toByteArray());
outStream.flush();
outStream.close();
}
}
[/code]
Hello Sir.
Thank you, it helps me a lot :))
Hello, actually i need do a QR Code scanner system ,also included generate QR Code function but i not familiar in Java. So can you teach me and help me?Please.
Sir/Madam i m new to java coding. i not getting how to run the above mentioned code.Please help me
i have face this error , So what should i do ? pls
Exception in thread “main” java.lang.NoSuchMethodError: com.google.zxing.Writer.encode(Ljava/lang/String;Lcom/google/zxing/BarcodeFormat;IILjava/util/Map;)Lcom/google/zxing/common/BitMatrix;
at net.glxn.qrgen.QRCode.createMatrix(QRCode.java:159)
at net.glxn.qrgen.QRCode.writeToStream(QRCode.java:155)
at net.glxn.qrgen.QRCode.stream(QRCode.java:134)
at ictti.CodeGen.main(CodeGen.java:14)
Hi,
How to download the generated QR code image.
Please guide me….
Hi,
i click more time but not get .some error page.(QR_Code_Servlet.zip (340 KB))
how to download this zip file.please guide me.