While working on an Android App, I had to integrate the Camera API. User can take a photo from App and process it. With Android the Photo that you click cannot be accessed until the media scanner runs and index it. It is possible to trigger programatically the Media Scanner in Android. Check the below code snippet:
import android.content.Intent;
import android.net.Uri;
import android.os.Environment;
...
...
sendBroadcast (
new Intent(Intent.ACTION_MEDIA_MOUNTED,
Uri.parse("file://" + Environment.getExternalStorageDirectory()))
);
...
Code language: Java (java)
Here we are using sendBroadcast method from Activity class to send a broadcast message to an Intent. For our need we have used ACTION_MEDIA_MOUNTED intent which will invoke the media scanner. Also note that we have passed the path (URI) of our external storage directory.
So in your app whenever you want to trigger Media scanner, simply invoke the above intent via broadcast message.
Following is a Demo App to achieve this.
Demo App
The App will be very simple. It will have a button “Trigger Media Scanner”. On its click we will invoke the above sendBroadcast() code to trigger media scanner.
Step 1: Create Basic Android Project in Eclipse
Create a Hello World Android project in Eclipse. Go to New > Project > Android Project. Give the project name as MediaScannerDemo and select Android Runtime 2.1 or sdk 7. I have given package name net.viralpatel.android.mediascanner
.
Once you are done with above steps, you will have a basic hello world Android App.
Step 2: Change the Layout
For our demo, we need simple layout. Just one button Trigger Image Scanner which does the job.
Open layout/main.xml in your android project and replace its content with following:
File: res/layout/main.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:gravity="center">
<Button
android:id="@+id/buttonMediaScanner"
android:layout_width="fill_parent"
android:text="Trigger Media Scanner"
android:textSize="25dp"
android:layout_height="100dp"></Button>
</LinearLayout>
Code language: HTML, XML (xml)
The UI is very simply. One LinearLayout to organize the button and one button. Note the id for button buttonMediaScanner
which we will use in our Java code.
Step 3: Android Java Code to trigger Image Scanner Intent
Open MediaScannerDemoActivity
class and add following code in OnCreate()
method.
package net.viralpatel.android.mediascanner;
import android.app.Activity;
import android.content.Intent;
import android.net.Uri;
import android.os.Bundle;
import android.os.Environment;
import android.view.View;
import android.view.View.OnClickListener;
import android.widget.Button;
import android.widget.Toast;
public class MediaScannerDemoActivity extends Activity {
/** Called when the activity is first created. */
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
Button buttonMediaScanner = (Button) findViewById(R.id.buttonMediaScanner);
//Add onClick event on Media scanner button
buttonMediaScanner.setOnClickListener(new OnClickListener() {
@Override
public void onClick(View view) {
//Broadcast the Media Scanner Intent to trigger it
sendBroadcast(new Intent(Intent.ACTION_MEDIA_MOUNTED, Uri
.parse("file://"
+ Environment.getExternalStorageDirectory())));
//Just a message
Toast toast = Toast.makeText(getApplicationContext(),
"Media Scanner Triggered...", Toast.LENGTH_SHORT);
toast.show();
}
});
}
}
Code language: Java (java)
Thus in OnCreate()
method, we have added an OnClickListener
to button. In the listener class, we added logic to trigger media scanner and also a Toast message to show user that scanner has been triggered.
Screen shots of Android App
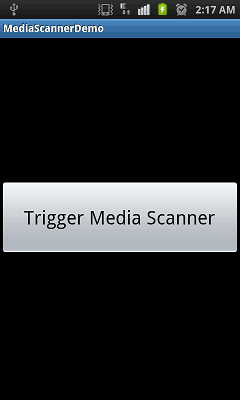
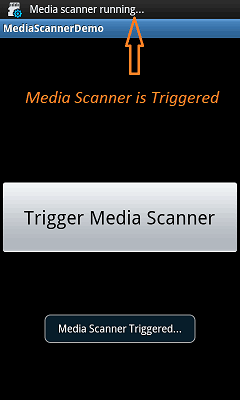
And that’s all! Just execute the app in Android emulator or real device and see following output.
On click of Trigger Media Scanner button, the media scanner is invoked which we can see in Title bar.
good apps
Toast only shown,It’s not working properly what you have given. I have used API Level10(AVD2.3.3)
Which format will it scan
Hi Manish,
When i run it on 4.3 Devices i got the following error
java.lang.SecurityException: Permission Denial: not allowed to send broadcast android.intent.action.MEDIA_MOUNTED
It’s just showing toast