If you are developing an Android app you may already fetching information from internet. While doing so there is a chance that internet connection is not available on users handset. Hence its always a good idea to check the network state before performing any task that requires internet connection. You might also want to check what kind of internet connection is available in handset. For example is wifi currently enabled? or is mobile data network is connected.
Check Internet Connection
Here is a simple code snippet that will help you identify what kind of internet connection a user has on her device. First we need following permission in order to access network state. Add following permission to your AndroidManifest.xml file. Permissions required to access network state:
<uses-permission android:name="android.permission.ACCESS_NETWORK_STATE" />
Code language: HTML, XML (xml)
Now check following utility class NetworkUtil
. It has method getConnectivityStatus
which returns an int constant depending on current network connection. If wifi is enabled, this method will return TYPE_WIFI
. Similarly for mobile data network is returns TYPE_MOBILE
. You got the idea!! There is also method getConnectivityStatusString
which returns current network state as a more readable string.NetworkUtil.java
package net.viralpatel.network;
import android.content.Context;
import android.net.ConnectivityManager;
import android.net.NetworkInfo;
public class NetworkUtil {
public static int TYPE_WIFI = 1;
public static int TYPE_MOBILE = 2;
public static int TYPE_NOT_CONNECTED = 0;
public static int getConnectivityStatus(Context context) {
ConnectivityManager cm = (ConnectivityManager) context
.getSystemService(Context.CONNECTIVITY_SERVICE);
NetworkInfo activeNetwork = cm.getActiveNetworkInfo();
if (null != activeNetwork) {
if(activeNetwork.getType() == ConnectivityManager.TYPE_WIFI)
return TYPE_WIFI;
if(activeNetwork.getType() == ConnectivityManager.TYPE_MOBILE)
return TYPE_MOBILE;
}
return TYPE_NOT_CONNECTED;
}
public static String getConnectivityStatusString(Context context) {
int conn = NetworkUtil.getConnectivityStatus(context);
String status = null;
if (conn == NetworkUtil.TYPE_WIFI) {
status = "Wifi enabled";
} else if (conn == NetworkUtil.TYPE_MOBILE) {
status = "Mobile data enabled";
} else if (conn == NetworkUtil.TYPE_NOT_CONNECTED) {
status = "Not connected to Internet";
}
return status;
}
}
Code language: Java (java)
You can use this utility class in your android app to check the network state of the device at any moment. Now this code will return you the current network state whenever the utility method is called. What if you want to do something in your android app when network state changes? Lets say when Wifi is disabled, you need to put your android app service to sleep so that it does not perform certain task. Now this is just one usecase. The idea is to create a hook which gets called whenever network state changes. And you can write your custom code in this hook to handle the change in network state.
Broadcast Receiver to handle changes in Network state
You can easily handle the changes in network state by creating your own Broadcast Receiver. Following is a broadcast receiver class where we handle the changes in network. Check onReceive()
method. This method will be called when state of network changes. Here we are just creating a Toast message and displaying current network state. You can write your custom code in here to handle changes in connection state.NetworkChangeReceiver.java
package net.viralpatel.network;
import android.content.BroadcastReceiver;
import android.content.Context;
import android.content.Intent;
import android.widget.Toast;
public class NetworkChangeReceiver extends BroadcastReceiver {
@Override
public void onReceive(final Context context, final Intent intent) {
String status = NetworkUtil.getConnectivityStatusString(context);
Toast.makeText(context, status, Toast.LENGTH_LONG).show();
}
}
Code language: Java (java)
Once we define our BroadcastReceiver, we need to define the same in AndroidMenifest.xml file. Add following to your menifest file.
<application ...>
...
<receiver
android:name="net.viralpatel.network.NetworkChangeReceiver"
android:label="NetworkChangeReceiver" >
<intent-filter>
<action android:name="android.net.conn.CONNECTIVITY_CHANGE" />
<action android:name="android.net.wifi.WIFI_STATE_CHANGED" />
</intent-filter>
</receiver>
...
</application>
Code language: HTML, XML (xml)
We defined our broadcast receiver class in menifest file. Also we defined two intent CONNECTIVITY_CHANGE
and WIFI_STATE_CHANGED
. Thus this will register our receiver for given intents. Whenever there is change in network state, android will fire these intents and our broadcast receiver will be called. Below is complete AndroidMenifest.xml file. AndroidMenifest.xml
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="net.viralpatel.network"
android:versionCode="1"
android:versionName="1.0" >
<uses-sdk
android:minSdkVersion="8"
android:targetSdkVersion="15" />
<uses-permission android:name="android.permission.ACCESS_NETWORK_STATE" />
<application
android:icon="@drawable/ic_launcher"
android:label="@string/app_name"
android:theme="@style/AppTheme" >
<receiver
android:name="net.viralpatel.network.NetworkChangeReceiver"
android:label="NetworkChangeReceiver" >
<intent-filter>
<action android:name="android.net.conn.CONNECTIVITY_CHANGE" />
<action android:name="android.net.wifi.WIFI_STATE_CHANGED" />
</intent-filter>
</receiver>
</application>
</manifest>
Code language: HTML, XML (xml)
Run this demo in android emulator or actual device. When Wifi is enabled, you’ll see a Toast message with message.
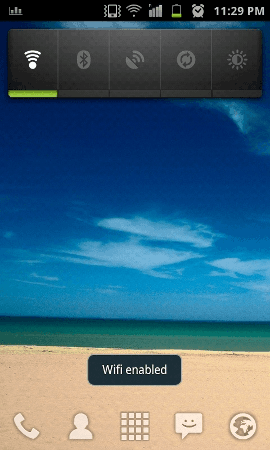
Now disable Wifi. The toast message will show you message that internet connection is not available.
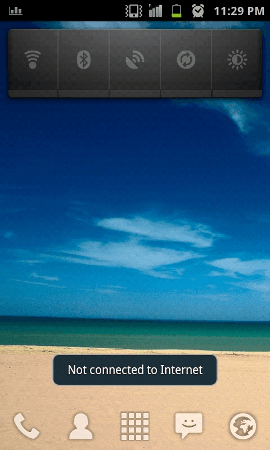
Now enable mobile data network. The same will be show in toast message as soon as you enable mobile data connection.
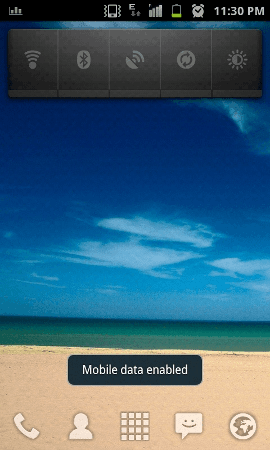
Download Source Code
Browse through the source code in following Git repository:
GitHub: https://github.com/viralpatel/android-network-change-detect-example
Download complete source code:
Download: android-network-change-detect-example.zip (376 KB)
Thanks a lot for the post !! It saved my day and lot of time :)
Thanks a lot
please repair Download link
very helpful, thanks.
thanks a lot.please post more other topic in android
Thanks a lot for your tutorial but I have an issue I have developed an app that can send my basic form information to google drive spreadsheet but I want to add a code where by if the user network is down it would pen the information and the moment network comes up it would send the information to the spreadsheet what can I do thanks.
What if the device is connected to a network and no internet access?
The first example on this page is a very bad piece of programming in my opinion. First you should check if you have a connection, then you should check what kind of connection it is. There are about a dozen different types of connections possible, and in this example only two are checked, and if it is neither one of them then it is concluded that there is no connection. Not so, what if you have a wired connection (ethernet) for instance? And you should only check what kind of connection you have after you concluded that there is a connection, which means that concluding that there is no connection because it is not mobile or wifi or what ever is wrong anyway.
Wired connection on a mobile device?
Thanks for all!!
Could you please explain? What ll happen, if i connect the device with ethernet?….
can u give .apk for this
How would I do this in my activity? would have to use the RegisterReceiver?
Hi this was really a cool tutorial but ive been thinking its not working when i try to run it from eclipse using the full source code thanks for the help
How to show a Dialog instead toast ?
Hey this code is not working I guess there is some prob can u help and cand u mail a fresh copy of it and its .apk
This wont work anymore, as of Kitkat! So, you need to explore other options :)
I am also currently working on it. Best of luck!
Hi!
I’ve tryied it on my samsung galagy s2 with android 4.1.2 and do not works. I’ve tryied it on avd with android 4.1.2 and do not works. I’ve tryied it on avd with android 4.4.2 and do not works. It works only with avd with android 2.3.3! Do you know why?
TNX
D!
Hi
Please replace your manifest file with below code
Then try it with version above 2.3, it will work
Hi
Try adding your MainActivity launcher code in your manifest file.
for example
”
”
Then try with version above 2.3
Hi
Please replace your manifest file with below code
?xml version=”1.0″ encoding=”utf-8″?>
Then try it with version above 2.3, it will work
Thanks for the tutorial.. I need a little help, I want different activities to be started as network status changes. To make it more simple, I want a specific activity to be started as long as the device is connected to internet but as it gets disconnected, it starts a new activity. Pls guide.
Thanks for this post! I have one question,could you please answer it?
Once wifi is connected I want to download file from server e.g tomcat , how can i do this?
I have file downloading code with me, I have tried this code on button click where it works properly i.e on button click i am able to download file from server, but how can i do this with broadcast receiver i.e when wi-fi is connected it should automatically download file from server?
it will only tell if u are connected to a network or not.It’ll not tell anything about the active internet connection.
Thank you very much…
Hi,
This tutorail is great to detect network connection enable/disable state and also for wifi to mobiledata switch event.
But i need to detect wifi to wifi switch event.is that possilbe and how.?
With thanks,
tanvir
I can’t run the project because there is no activity found.
Thank you very much…
Thanks for this tutorial, but I have a question.Will the toasts be shown when a user has changed wifi state of his/her mobile
sir it is displaying msg two time.
exmpl= connected date ,connected data
what should i do to it display msg only once.
thnks
smart coding…great tutorial…Thanks
hello how can i display retry and cancel message in Web View if internet connection lost ,and when connected to internet it should reload my page in same Web View app?
Hi.
This works fine. How can I make the application run this service from boot time?
These two lines gives me errors. Kindly assist me on this.
Works fine. Thanks for the info.
Hello, thanks a lot. Is there a way to detect background data restricted status for android?
A big thank you for this great article. it saved a lot of time.