Are you bugged of creating separate action classes for some common set of functionality in your Struts application? Feeling pain in managing all those hundreds of Action classes in your project? Don’t worry, cheers..!! DispatchAction is for you. DispatchAction is one of the Struts built-in action that provides a mechanism that facilitates having a set of related functionality in a single action instead of creating separate independent actions for each function. Let us create a sample example that uses DispatchAction and implement certain functionality of our project. Before that, I assume you have working knowledge of Struts application. If you don’t, Read this tutorial about A Hello World Struts project and get some basics about Struts application. Also, I assume you have already got a Struts application and you want to add Dispatch Action into it. So let us start with our example.
Click the link Create User or Delete User etc. and see the output.
Step 1: Create DispatchAction class file.
Create an Action class called UserManagementAction and extend it with class org.apache.struts.actions.DispatchAction. Copy following code into it.package net.viralpatel.struts.helloworld.action;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import org.apache.struts.action.ActionForward;
import org.apache.struts.action.ActionMapping;
import org.apache.struts.actions.DispatchAction;
public class UserManagementAction extends DispatchAction {
public ActionForward create(ActionMapping mapping, ActionForm form,
HttpServletRequest request, HttpServletResponse response)
throws Exception {
request.setAttribute("message", "User created successfully");
return mapping.findForward("success");
}
public ActionForward delete(ActionMapping mapping, ActionForm form,
HttpServletRequest request, HttpServletResponse response)
throws Exception {
request.setAttribute("message", "User deleted successfully");
return mapping.findForward("success");
}
public ActionForward update(ActionMapping mapping, ActionForm form,
HttpServletRequest request, HttpServletResponse response)
throws Exception {
request.setAttribute("message", "User updated successfully");
return mapping.findForward("success");
}
public ActionForward block(ActionMapping mapping, ActionForm form,
HttpServletRequest request, HttpServletResponse response)
throws Exception {
request.setAttribute("message", "User blocked successfully");
return mapping.findForward("success");
}
}
Code language: Java (java)
In above code, we have created separate methods (create(), delete(), update() and block()) for each functionality. Also note that the method signature of these methods are exactly similar to the execute() method of Action class file.public ActionForward delete(ActionMapping mapping, ActionForm form,
HttpServletRequest request, HttpServletResponse response)
throws Exception { }
Code language: Java (java)
Step 2: Create a mapping for action in struts-config.xml file.
Open your struts-config.xml and copy following action mapping entry in it.<action path="/user" parameter="parameter"
type="net.viralpatel.struts.helloworld.action.UserManagementAction">
<forward name="success" path="/UserSuccess.jsp" />
<forward name="failure" path="/UserSuccess.jsp" />
</action>
Code language: HTML, XML (xml)
We have added an extra attribute in <action> tag, parameter=”parameter”. DispatchAction will read a request parameter called “parameter” and its value will decide the method to be called. Suppose you have a request parameter “parameter” with value “create”, Dispatch Action will call create() method from your Action file.Step 3: Create JSPs for viewing the application.
Create two JSP files UserManagement.jsp and UserSuccess.jsp and copy following code into it. UserManagement.jsp will display a menu for selecting action to be taken. UserSuccess.jsp will just print the appropriate action taken by the Action class.UserManagement.jsp
<%@taglib uri="http://jakarta.apache.org/struts/tags-html" prefix="html"%>
<html>
<head>
<title>Dispatch Action Example - viralpatel.net</title>
</head>
<body>
<h2>User Management (Dispatch Action Example)</h2>
<html:link href="user.do?parameter=create">Create User</html:link>
|
<html:link href="user.do?parameter=delete">Delete User</html:link>
|
<html:link href="user.do?parameter=update">Update User</html:link>
|
<html:link href="user.do?parameter=block">Block User</html:link>
</body>
</html>
Code language: HTML, XML (xml)
UserSuccess.jsp
<%@taglib uri="http://jakarta.apache.org/struts/tags-html" prefix="html"%>
<html>
<head>
<title>Dispatch Action Example - viralpatel.net</title>
</head>
<body>
<h3>User Message (Dispatch Action Example)</h3>
<center>
<font color="blue"><h3><%= request.getAttribute("message") %></h3></font>
<center>
</body>
</html>
Code language: HTML, XML (xml)
In UserManagement.jsp, we have created links using <html:link> tag and passed a parameter “parameter” with values create, delete, block etc. This value is fetched by the Dispatch Action and is used to call corresponding method in Action class. Thus if we click Update User link, a parameter update will be passed to the action class and corresponding update() method will be called by framework.Step 4: Run the application.
Compile and Run the project using Eclipse or Ant and open UserManagement.jsp file in your favorite browser.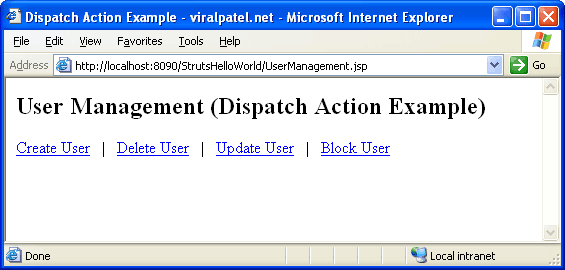
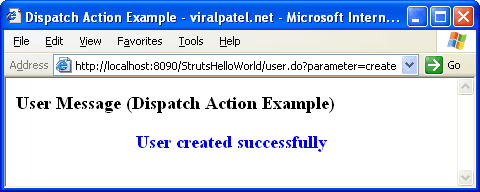
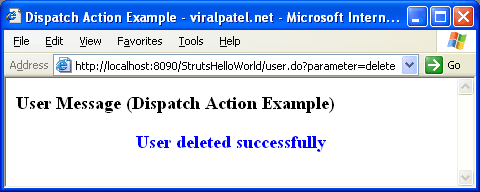
Excelente manual!!! Muchas gracias
Thank’s
@Carlos: Thanks for the good words :) Do read other articles about Struts and also feel free to subscribe for the newsletter.
Nice Tutorial,Very Good
If we have a from in which we are trying to use Dispatch action, What is the best way of implementing it. I have used the parameter in action tag of a Form, something like, action=’dynaAction.do?param=ParamValue’. depends on the action selected, we can change the URL for the form. Do you have any other approach in doing this.
Thanks,
Kumar
hello viral
my question is that ,cannot we use buttons instead of html-link ??…..
b’coz when I m using buttons instead of links I m getting following error
” Request[/ClientForm] does not contain handler parameter named ‘parameter’. This may be caused by whitespace in the label text”….
Hi Neelam,
The possible reason of error in case of using button instead of link is that it is not able to get parameter to decided which method to trigger in Struts. Create a field (hidden or select box) in your form with name=”parameter” and set its value to method name that you want to call.
Hope this will solve the issue.
hi viral
I have tried this using
**********jsp…************
***********struts-config…*************
********ApplicatonResources…***********
label.save=save
*********************ClientAction_Buttons.java***************************
protected Map getKeyMethodMap() {
Map map = new HashMap();
map.put(“label.add”, “add”);
map.put(“label.edit”, “edit”);
map.put(“label.search”, “search”);
map.put(“label.save”, “save”);
return map;
}
public ActionForward add(
ActionMapping mapping,
ClientForm form,
HttpServletRequest request,
HttpServletResponse response) throws Exception {
System.out.println(“You are in add function.”);
return mapping.findForward(“add”);
}
**************
but now I m getting the following error
javax.servlet.ServletException: DispatchMapping[/ClientForm] does not define a handler property..
can you please tell me what I doing wrong here???
Regards
Neelam
“javax.servlet.ServletException: DispatchMapping[/ClientForm] does not define a handler property..
can you please tell me what I doing wrong here???”
Your add() method is having a parameter of type ClientForm, Replace the ClientForm with ActionForm and check if it works.
I think this is not a good solution. In some situations it is not useful and cannot be implemented. How can we extend both ActionSupport and DispatchAction. In modern OOP, a class should be have one and only one superclass.
Hi viral,i am daily visitor of u r website,u r posts are very good and i am following the same.Me working as a s/w professional in a small organization.could you pls post one sample project with dao,vo,action,domain layer.
Hi Neelam,
I guess, now you have used a hidden field named “parameter” on the jsp, but you have not
created a property by the name “parameter” on the corresponding form for that jsp.
if we use dispatch action how many formbean(Action Forms) classes we have to create.
if i want to put a specific method of dispatch action in thread, then what will i have to do .
Go through this link :
http://www.raistudies.com/struts-1/dispatchaction/
Realy good illustration of DispatchAction with real life example and code.
nice tutorial. this is exactly what i required.
@ Viral :) Excellent Work! Very Good!! I Easily Understood the concept.. thank You Viral Patel
Hello viral
I would like to know what to do in case i have three buttons add, update , delete..
Then how do i pass a parameter to action class.
Well said.But my doubt is ActionForm need or not?
I have done the same prog. with buttons and using the scope but problem is that its not showing any result
—————————————————————————————————————-
Student Id :
Student Name :
————————————————————————————————————————-
———————————————————————————————————————–
public class CustomerDispatchForm extends org.apache.struts.action.ActionForm {
protected String sid;
protected String name;
public String getSid() {
return sid;
}
public void setSid(String sid) {
this.sid = sid;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
}
—————————————————————————————————————————
public class CustomerDispatchAction extends DispatchAction {
String sid;
String name;
public ActionForward saveCustomer(ActionMapping mapping,ActionForm form,HttpServletRequest request,HttpServletResponse response) {
CustomerDispatchForm fm=(CustomerDispatchForm) form;
sid=fm.getSid();
name=fm.getName();
request.setAttribute(“Student ID”, sid);
request.setAttribute(“Student Name”, name);
return mapping.findForward(“success”);
}
public ActionForward deleteCustomer(ActionMapping mapping,ActionForm form,HttpServletRequest request,HttpServletResponse response) {
CustomerDispatchForm fm=(CustomerDispatchForm) form;
sid=fm.getSid();
name=fm.getName();
request.removeAttribute(“sid”);
request.removeAttribute(“name”);
return mapping.findForward(“success”);
}
}
————————————————————————————————————————–
———————————————————————————————————————-
action
org.apache.struts.action.ActionServlet
config
/WEB-INF/struts-config.xml
debug
2
detail
2
2
action
*.do
30
Input.jsp
/WEB-INF/struts-bean.tld
/WEB-INF/struts-bean.tld
/WEB-INF/struts-html.tld
/WEB-INF/struts-html.tld
/WEB-INF/struts-logic.tld
/WEB-INF/struts-logic.tld
/WEB-INF/struts-nested.tld
/WEB-INF/struts-nested.tld
/WEB-INF/struts-tiles.tld
/WEB-INF/struts-tiles.tld
======================================================================
Help Me to understand the error.
Nice one… Saved my time….
Hi viral, when I try this example , I get the 404- Resource not found error, what could be the reason ?
Hi Viral
Thanks a lot!
I have one query. Can We hide method name in URL?
I tried hard!but not success-ed!
Thanks in Advance.
Kailash
Hi Viral,
I am a new bee in struts. i am using 1.3 version of struts.
Can we have multiple execute methods in one acton class in struts if we are not using DispatchAction mechanism ?
What will happen if we try to use multiple execute methods ?
Your help is appreciated.
Awaiting for your answer ASAP.
Regards,
Vijay
Hi Viral,
thank you soo much for this super manual.. Its very easy to understand and it is very clear.
Hi Viral
I m getting the following issue is I click on any of the link on the first page load
Create User | Delete User | Update User | Block User
url is: http://localhost:8080/DA/UserManagement.jsp
type Status report
message Servlet action is not available
description The requested resource is not available.
hi viral ,
I have used DispatchActon class and i m getting error as DispatchMapping[/getEmp] does not define a handler property.Here is my code.Please suggest smthing to solve this error.
index.jsp
dispatchAction.java
struts-config.xml
displayTest.jsp
thanks in advanced
I’m new to struts while creating an appln I met with some issue I got cleared with this link thanks a lot dear
hii…I want to know that how to call create ,edit and delete in action by dispatcg action?
Hi,
thanks for this. this helped me to explain same functionality in interview .
regards
vinodh
i need two different form in one jsp page,which has only one front controller servlet , and two different beans .. in struts 1. . plz help me
The below code is returning null .
i believe you need to store the ‘message’ to some variable and print it. correct me if i am wrong.
Hi Viral,
the below Code is not working , “message” is printing as null.
I used like this,now it is working , kindly reckeck