A Custom tag is a user defined JSP language element. When a JSP page containing custom tag is translated into a Servlet, the tag is converted to operations on an object called tag handler. The Web container then invokes those operations when the JSP page’s servlet is executed. It speeds up web application development because of code reuse feasibility. Custom tags can access all the objects available in JSP pages. Custom tags can modify the response generated by the calling page. Custom tags can be nested. Custom tag library consists of one or more Java classes called Tag Handlers and an XML tag library descriptor file (tag library). A class which has to be a tag handler needs to implement Tag interface or IterationTag interface or BodyTag interface or it can also extend TagSupport class or BodyTagSupport class. All the classes that support custom tags are present inside a package called javax.servlet.jsp.tagext. Let us create a custom tag which will does substring operation on given input. For this we will first create our Tag Handler class. Create a class called SubstrTagHandler and copy/paste following code in it.
package net.viralpatel.jsp.custom.taglib;
import java.io.IOException;
import javax.servlet.jsp.JspException;
import javax.servlet.jsp.JspWriter;
import javax.servlet.jsp.tagext.TagSupport;
public class SubstrTagHandler extends TagSupport {
private String input;
private int start;
private int end;
@Override
public int doStartTag() throws JspException {
try {
//Get the writer object for output.
JspWriter out = pageContext.getOut();
//Perform substr operation on string.
out.println(input.substring(start, end));
} catch (IOException e) {
e.printStackTrace();
}
return SKIP_BODY;
}
public String getInput() {
return input;
}
public void setInput(String input) {
this.input = input;
}
public int getStart() {
return start;
}
public void setStart(int start) {
this.start = start;
}
public int getEnd() {
return end;
}
public void setEnd(int end) {
this.end = end;
}
}
Code language: Java (java)
In above code we have created three attributes: input, start and end. These are the inputs that we will get when the custom tag will be invoked from a JSP file. Also note that, these attributes have getter and setter methods which will be used to set the property values. Now we will create the tag descriptor file also called TLD. Create a file called SubstrDescriptor.tld in WEB-INF directory of your web project and copy / paste following content in it.<?xml version="1.0" encoding="UTF-8"?>
<taglib>
<tlibversion>1.0</tlibversion>
<jspversion>1.1</jspversion>
<shortname>substr</shortname>
<info>Sample taglib for Substr operation</info>
<uri>https://www.viralpatel.net/jsp/taglib/substr</uri>
<tag>
<name>substring</name>
<tagclass>net.viralpatel.jsp.custom.taglib.SubstrTagHandler</tagclass>
<info>Substring function.</info>
<attribute>
<name>input</name>
<required>true</required>
</attribute>
<attribute>
<name>start</name>
<required>true</required>
</attribute>
<attribute>
<name>end</name>
<required>true</required>
</attribute>
</tag>
</taglib>
Code language: HTML, XML (xml)
In above tld file, <tag> is used to define a custom tag. Each new tag will have its own tag handler class which we specify in <tagclass> tag. Also <name> tag in <tag> represents the tag name that we use in jsp file. We have provided the three attributes with this tag: input, start and end. input is the String whose sub string needs to be parsed. start is the start index and end is the end index. We have just created our first Custom Taglib for JSP. Now let us use the custom taglib in a JSP file. For this create index.jsp in your web application (if it exists, modify it and add this code) and add following code in it. <required> true make these attribute mandatory.<%@taglib prefix="test" uri="/WEB-INF/SubstrDescriptor.tld"%>
<html>
<head>
<title>JSP Custom Taglib example: Substr function</title>
</head>
<body>
SUBSTR(GOODMORNING, 1, 6) is
<font color="blue">
<test:substring input="GOODMORNING" start="1" end="6"/>
</font>
</body>
</html>
Code language: HTML, XML (xml)
Following is the screenshot of the output. 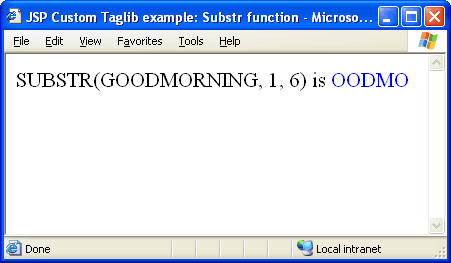
I tried your example but then it fails since it does not recognise the url part in the tld file.
include that .tld in ur web.xml
You should include this library http://tomcat.apache.org/download-taglibs.cgi , in your [WEB-INF/lib] directory then add the jar in the build path of your project…..afterward it should work!!
thank you for the explanation I wish if you put more examples, some real examples i mean the ones you usually use in your code, because i cannot understand exactly why i should use it when I can use EL function
Its gud to provide such facility.thank u.
thanks.
I tried your example and it works perfectly fine for me. Thanks for posting this.
This is really a very good site. The example given is very fine and it worked very well.
Thank you very much :)
This is very clear and helpful. Thanks for posting :)
it is very clear:)
Very Good and quite simple implementation. Thanks Dude
Thanks…:-)
its easy to understand..
Thanks Viral. The tutorial was quite helpful.
Thank you very much viral for providing useful example
Awesome..You explained it to easily…Keep up the good work!
Thanks :D
Thanks for the quickly and very clear explanation of custom tags in a very short article.
good one !
Thanks
Really very good example.
nice example….. very clear.
good example……my totaly concept is clear about custom tag
nice one.. its very useful
Thank you so much. It worked without any changes .
Very nice and simple example.
Thank you so much. Very nice and easy to understand tutorial.
This is the 2nd blog, of your site I actually read through.
And yet I actually enjoy this specific 1, “Tutorial: Create JSP custom tag library | JSP Custom Tag
| Taglib” the very best. Thanks -Bobbie withprimesolarwindowshades.
com/window-shades
Thanks
how do i create jsp textbox custom tag?
Nice exampal, this really help ful for new tag library development thank lot for nice work viral
Can you explain what is the difference between
and
How is interpreted – for example namespace?
like can we create the tags in spring mvc … i need to create the calendar tag how can i… or is any other way to use it.
Can you please answer to this question. http://stackoverflow.com/questions/17136255/unable-to-access-nested-jsp-tags-in-freemarker
Nice Example. Easy to understand. Thanks for posting.
Thanks for the basic example
when you have defined URI then you should provide same URI in JSP page. Nevertheless it will work .But then what ‘s use of giving URI when you use relative path of TLD file ?
It seems unnecessary
why are you asking to copy paste ,Instead you have been used comments to explain what exactly is happening
Thanks, nice post
I got mine to work easily, the first time by following your example. Thank you for providing a complete example.
very nicee………….
Thanks .very nice example ……….
This is very helpful for all…………………. Nice
Thanks dude… your tutorials are really easy to understand and implement. This is a really good example.
I am getting an error in my code. can you answer my this question.
http://stackoverflow.com/questions/22758863/unable-to-find-setter-method-for-attribute-in-jstl?noredirect=1#comment34693594_22758863
Very helpful article, I could quickly understand how/what tags are for.
Hi Viral ,
Very nice you have explain Custom Tag Example.
i understood very clearly , Thanks a lot !!!
can u answer my this question ?
http://stackoverflow.com/questions/23284948/custom-tag-is-not-working-properly
Thanks! this is simple, clear example and can be duplicated.
Thanks, it helped!
Thank You for the example..!!
Very good tutorial.finally i learn about jsp custom tag here thank u very much……